TensorFlow实现目标识别代码
时间: 2024-01-19 18:44:54 浏览: 33
以下是使用TensorFlow实现目标识别的代码示例:
首先,需要安装TensorFlow和其他必要的库。可以使用以下命令安装:
```
pip install tensorflow
pip install pillow
pip install matplotlib
pip install numpy
```
然后,可以使用以下代码加载训练集和测试集的图像数据:
```python
import tensorflow as tf
import matplotlib.pyplot as plt
import numpy as np
from PIL import Image
# Load training and testing datasets
train_images = np.load('train_images.npy')
train_labels = np.load('train_labels.npy')
test_images = np.load('test_images.npy')
test_labels = np.load('test_labels.npy')
```
接下来,需要定义模型并进行训练。可以使用以下代码:
```python
# Define the model
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, (3, 3), activation='relu', input_shape=(28, 28, 1)),
tf.keras.layers.MaxPooling2D((2, 2)),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(10, activation='softmax')
])
# Compile the model
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# Train the model
model.fit(train_images, train_labels, epochs=10)
```
最后,可以使用以下代码评估模型在测试集上的表现:
```python
# Evaluate the model on the test set
test_loss, test_acc = model.evaluate(test_images, test_labels, verbose=2)
print('\nTest accuracy:', test_acc)
```
以上是使用TensorFlow实现目标识别的基本代码示例,具体实现可以根据需求进行修改和优化。
相关推荐
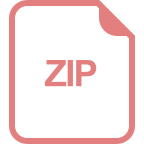
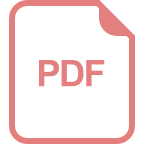
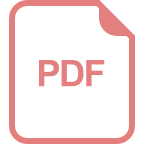














