C#设计一个“我的数学类mymath”,内含以下静态方法: 1.求一元二次函数(y=ax2+bx+c)值的静态方法,参数自行考虑设计,方法返回y的值; 2.输出一维数组各元素的方法,一个元素输出一行,并输出元素下标,输出示例:“下标为0的元素值为:90”
时间: 2024-03-16 08:41:13 浏览: 30
好的,以下是 C# 设计的“我的数学类mymath”:
```csharp
using System;
public class MyMath
{
public static double QuadraticFunction(double a, double b, double c, double x)
{
return a * x * x + b * x + c;
}
public static void PrintArray(int[] arr)
{
for (int i = 0; i < arr.Length; i++)
{
Console.WriteLine("下标为" + i + "的元素值为:" + arr[i]);
}
}
}
```
第一个静态方法 `QuadraticFunction` 求一元二次函数的值,参数为 `a`、`b`、`c` 和 `x`,返回 `y` 的值。第二个静态方法 `PrintArray` 输出一维数组各元素的值,参数为一个整型数组 `arr`。
相关问题
创建MyMath.py文件,编写MyMath类,编写以下对象方法:pow(xy) 返回x的y次幂的
代码实现如下:
```python
class MyMath:
def pow(self, x, y):
return x ** y
```
在上面的代码中,我们定义了一个名为`MyMath`的类,其中有一个名为`pow`的方法。该方法接收两个参数`x`和`y`,并返回`x`的`y`次幂的结果。
要使用这个类,需要将上面的代码保存到一个名为`MyMath.py`的文件中,并在需要使用这个类的地方导入它。例如:
```python
from MyMath import MyMath
m = MyMath()
result = m.pow(2, 3)
print(result) # 输出8
```
创建MyMath.py文件,编写MyMath类,编写以下对象方法:fioor(x) 返回不大于x的最大整数
好的,以下是MyMath.py文件中的代码实现:
```python
class MyMath:
def floor(self, x):
return int(x // 1)
```
这里定义了一个MyMath类,其中有一个floor方法,其作用是返回不大于x的最大整数。我们使用了Python内置的int函数来实现这个功能。注意,这个方法必须要通过实例化MyMath类来调用,例如:
```python
math = MyMath()
print(math.floor(3.14)) # 输出 3
print(math.floor(5.99)) # 输出 5
```
相关推荐
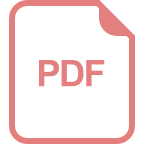
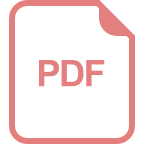













