#include "movie.h" #include <QString> #include <fstream> using std::string; //创建电影类 Movie::Movie() {} Movie::Movie(string name, string director, string actor, string time) { this->name = name;//电影名称 this->director = director;//导演 this->actor = actor;//演员 this->time = time;//上映时间 } //搜索匹配关键字 auto Movie::isMatched(string name, string director, string actor, string time) -> bool { bool ret = true; if (this->name.find(name) == string::npos) ret = false; if (this->actor.find(actor) == string::npos) ret = false; if (this->director.find(director) == string::npos) ret = false; if (this->time.find(time) == string::npos) ret = false; return ret; } //把电影对象转化成字符串 auto Movie::toString() const -> string { char buffer[1024]; sprintf(buffer, "%30s%30s%30s%30s", this->name.c_str(), this->director.c_str(), this->actor.c_str(), this->time.c_str()); return string(buffer); } //重载输入流运算符,将数据从文件中读取 auto operator>>(std::ifstream& is, Movie& m) -> std::ifstream& { is >> m.name >> m.director >> m.actor >> m.time; return is; } //重载输入流运算符,将数据写入文件中 auto operator<<(std::ofstream& os, const Movie& m) -> std::ofstream& { os << m.toString(); return os; } auto Movie::toQStringList() const -> QStringList { QStringList ret; ret.append(QString::fromStdString(this->name)); ret.append(QString::fromStdString(this->director)); ret.append(QString::fromStdString(this->actor)); ret.append(QString::fromStdString(this->time)); return ret; } auto Movie::operator==(const Movie& another) -> bool { if (another.name != this->name && another.name != "*") return false; if (another.director != this->director && another.director != "*") return false; if (another.actor != this->actor && another.actor != "*") return false; if (another.time != this->time && another.time != "*") return false; return true; } 什么意思
时间: 2024-04-26 13:25:44 浏览: 12
这段代码定义了一个电影类 `Movie`,包含电影名称、导演、演员、上映时间等信息,并提供了一些方法来操作这些信息。
具体来说:
- 构造函数 `Movie::Movie()` 和 `Movie::Movie(string name, string director, string actor, string time)` 用于创建电影对象,可以传入电影名称、导演、演员、上映时间等信息。
- 方法 `Movie::isMatched(string name, string director, string actor, string time)` 用于判断电影对象是否匹配给定的关键字,即电影名称、导演、演员、上映时间是否包含给定的字符串。
- 方法 `Movie::toString() const` 将电影对象转化成字符串。
- 运算符重载 `operator>>` 和 `operator<<` 用于将电影对象从文件中读取和写入文件中。
- 方法 `Movie::toQStringList() const` 将电影对象转化成 `QStringList`,用于在 Qt 的界面中显示电影信息。
- 运算符重载 `operator==` 用于比较两个电影对象是否相等,即电影名称、导演、演员、上映时间是否相等。其中,如果电影名称、导演、演员、上映时间有一个不匹配,则返回 false。如果其中一个属性值为 "*",则表示该属性不进行比较,即忽略该属性。
相关问题
QString 转std::vector<std::string>
QString是Qt框架中的一个类,用于处理字符串。如果你想将QString转换为std::vector<std::string>,可以按照以下步骤进行操作:
1. 首先,将QString转换为std::string。可以使用QString的toStdString()函数来实现这一步骤。
2. 接下来,将std::string转换为std::vector<std::string>。可以使用std::istringstream和std::getline函数来实现这一步骤。具体步骤如下:
```cpp
#include <QString>
#include <vector>
#include <string>
#include <sstream>
std::vector<std::string> QStringToVector(const QString& qstr) {
std::vector<std::string> result;
std::string str = qstr.toStdString();
std::istringstream iss(str);
std::string line;
while (std::getline(iss, line)) {
result.push_back(line);
}
return result;
}
```
这样,你就可以将QString转换为std::vector<std::string>了。
QVector<QString>和std::vector<cv::string>互转
要将QVector<QString>和std::vector<cv::string>互相转换,你可以使用以下方法:
1. 将QVector<QString>转换为std::vector<cv::string>:
- 首先创建一个空的std::vector<cv::string>列表。
- 然后遍历QVector<QString>,将每个QString元素转换为cv::string,并将其添加到std::vector<cv::string>中。
- 最后,你就可以使用std::vector<cv::string>了。
2. 将std::vector<cv::string>转换为QVector<QString>:
- 首先创建一个空的QVector<QString>列表。
- 然后遍历std::vector<cv::string>,将每个cv::string元素转换为QString,并将其添加到QVector<QString>中。
- 最后,你就可以使用QVector<QString>了。
注意,在进行转换之前,确保你已经包含了所需的头文件,并且在使用cv::string之前,你也应该使用了命名空间cv。
请注意,这里的转换方法是一种通用的方法,可以用于其他类型的向量或列表的转换。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* [vector2d:Ruby库(和gem),用于处理二维向量](https://download.csdn.net/download/weixin_42181693/15756579)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
- *2* *3* [趣谈implicit instantiation of undefined template](https://blog.csdn.net/liunanya/article/details/129596469)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
相关推荐
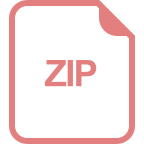
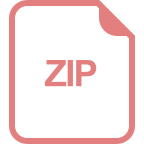
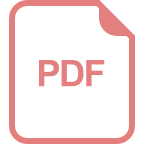













