<el-select clearable filterable v-model.lazy="scope.row.supplier_id"> <el-option v-for="sup in supList" :key="sup.supplier_id" :label="sup.supplier_name" :value="sup.supplier_id"> </el-option> </el-select>如何避免for循环导致的卡顿
时间: 2023-07-06 09:30:38 浏览: 62
在 Vue.js 中,使用 `v-for` 渲染大量数据时,可能会导致页面卡顿,因为每次数据更新都会重新渲染整个列表。为了避免这种情况,可以采用以下方式:
1. 使用 `v-for` 的 `key` 属性,可以提高性能和渲染速度,它会告诉 Vue.js 哪些元素需要被更新,哪些需要被替换,从而避免无谓的 DOM 操作。
2. 使用 `v-once` 指令,可以让 Vue.js 只渲染一次,不会随着数据的变化而重新渲染。但是,这种方式只适用于静态数据,对于动态数据无法使用。
3. 使用分页或虚拟滚动技术,可以将大量数据分成多个页面或虚拟滚动区域,每次只渲染当前页面或可见区域内的数据,从而避免一次性渲染大量数据。
下面是一个使用虚拟滚动的示例代码:
```vue
<template>
<div class="virtual-scroll-container" ref="container">
<div class="virtual-scroll-content" :style="{ height: contentHeight + 'px' }" ref="content">
<div class="virtual-scroll-item" v-for="(sup, index) in visibleData" :key="sup.supplier_id" :style="{ top: itemHeight * index + 'px' }">
<el-option :label="sup.supplier_name" :value="sup.supplier_id"></el-option>
</div>
</div>
</div>
</template>
<script>
export default {
data() {
return {
supList: [
{ supplier_id: 1, supplier_name: '供应商1' },
{ supplier_id: 2, supplier_name: '供应商2' },
{ supplier_id: 3, supplier_name: '供应商3' },
// ... 大量数据
],
visibleData: [],
itemHeight: 36, // 每个项的高度
contentHeight: 0, // 内容区域的高度
viewportHeight: 200, // 可见区域的高度
scrollTop: 0, // 滚动条的位置
};
},
mounted() {
this.contentHeight = this.supList.length * this.itemHeight;
this.updateVisibleData();
this.$refs.container.addEventListener('scroll', this.handleScroll);
},
beforeDestroy() {
this.$refs.container.removeEventListener('scroll', this.handleScroll);
},
methods: {
updateVisibleData() {
const scrollTop = this.scrollTop;
const start = Math.floor(scrollTop / this.itemHeight);
const end = start + Math.ceil(this.viewportHeight / this.itemHeight) + 1;
this.visibleData = this.supList.slice(start, end);
},
handleScroll(event) {
this.scrollTop = event.target.scrollTop;
this.updateVisibleData();
},
},
};
</script>
```
在上面的代码中,我使用虚拟滚动技术来渲染大量数据。首先,计算出内容区域的高度,然后根据可见区域的高度和每个项的高度,计算出可见的项数,并根据滚动条的位置,计算出需要显示的数据。在滚动条滚动时,更新可见的数据。这样,即使有大量数据,也只会渲染可见区域内的数据,从而提高页面的性能和渲染速度。
相关推荐
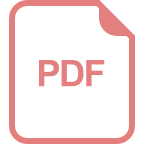
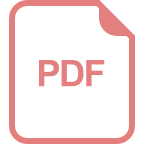








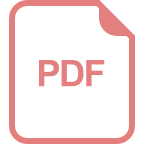
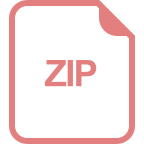
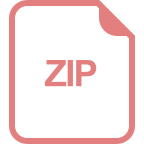
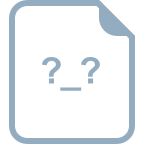
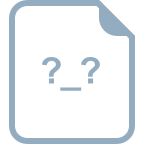