编写一个安卓的员工管理系统代码
时间: 2024-02-11 16:06:49 浏览: 19
这是一个简单的员工管理系统,包括添加、删除、修改和查询员工信息的功能。以下是代码:
MainActivity.java
```java
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
import androidx.appcompat.app.AppCompatActivity;
import java.util.ArrayList;
import java.util.List;
public class MainActivity extends AppCompatActivity {
private EditText editName, editAge, editPosition, editSalary;
private Button btnAdd, btnDelete, btnUpdate, btnSearch;
private List<Employee> employeeList = new ArrayList<>();
private int currentIndex = -1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
editName = findViewById(R.id.edit_name);
editAge = findViewById(R.id.edit_age);
editPosition = findViewById(R.id.edit_position);
editSalary = findViewById(R.id.edit_salary);
btnAdd = findViewById(R.id.btn_add);
btnDelete = findViewById(R.id.btn_delete);
btnUpdate = findViewById(R.id.btn_update);
btnSearch = findViewById(R.id.btn_search);
btnAdd.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
addEmployee();
}
});
btnDelete.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
deleteEmployee();
}
});
btnUpdate.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
updateEmployee();
}
});
btnSearch.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
searchEmployee();
}
});
}
private void addEmployee() {
String name = editName.getText().toString();
int age = Integer.parseInt(editAge.getText().toString());
String position = editPosition.getText().toString();
double salary = Double.parseDouble(editSalary.getText().toString());
Employee employee = new Employee(name, age, position, salary);
employeeList.add(employee);
currentIndex = employeeList.size() - 1;
Toast.makeText(this, "员工添加成功!", Toast.LENGTH_SHORT).show();
}
private void deleteEmployee() {
if (currentIndex >= 0 && currentIndex < employeeList.size()) {
employeeList.remove(currentIndex);
currentIndex = -1;
Toast.makeText(this, "员工删除成功!", Toast.LENGTH_SHORT).show();
} else {
Toast.makeText(this, "没有可删除的员工!", Toast.LENGTH_SHORT).show();
}
}
private void updateEmployee() {
if (currentIndex >= 0 && currentIndex < employeeList.size()) {
String name = editName.getText().toString();
int age = Integer.parseInt(editAge.getText().toString());
String position = editPosition.getText().toString();
double salary = Double.parseDouble(editSalary.getText().toString());
Employee employee = new Employee(name, age, position, salary);
employeeList.set(currentIndex, employee);
Toast.makeText(this, "员工信息更新成功!", Toast.LENGTH_SHORT).show();
} else {
Toast.makeText(this, "没有可更新的员工!", Toast.LENGTH_SHORT).show();
}
}
private void searchEmployee() {
String name = editName.getText().toString();
for (int i = 0; i < employeeList.size(); i++) {
Employee employee = employeeList.get(i);
if (employee.getName().equals(name)) {
currentIndex = i;
editAge.setText(String.valueOf(employee.getAge()));
editPosition.setText(employee.getPosition());
editSalary.setText(String.valueOf(employee.getSalary()));
Toast.makeText(this, "员工信息查询成功!", Toast.LENGTH_SHORT).show();
return;
}
}
Toast.makeText(this, "没有查询到该员工的信息!", Toast.LENGTH_SHORT).show();
}
}
```
Employee.java
```java
public class Employee {
private String name;
private int age;
private String position;
private double salary;
public Employee(String name, int age, String position, double salary) {
this.name = name;
this.age = age;
this.position = position;
this.salary = salary;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public String getPosition() {
return position;
}
public double getSalary() {
return salary;
}
}
```
activity_main.xml
```xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:padding="16dp">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="员工信息管理系统"
android:textSize="24sp"
android:textStyle="bold" />
<EditText
android:id="@+id/edit_name"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:hint="姓名" />
<EditText
android:id="@+id/edit_age"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:inputType="number"
android:hint="年龄" />
<EditText
android:id="@+id/edit_position"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:hint="职位" />
<EditText
android:id="@+id/edit_salary"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:inputType="numberDecimal"
android:hint="薪水" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:orientation="horizontal">
<Button
android:id="@+id/btn_add"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="添加" />
<Button
android:id="@+id/btn_delete"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:text="删除" />
<Button
android:id="@+id/btn_update"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:text="更新" />
<Button
android:id="@+id/btn_search"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:text="查询" />
</LinearLayout>
</LinearLayout>
```
此代码仅供参考,可能需要根据实际情况进行修改和调整。
相关推荐
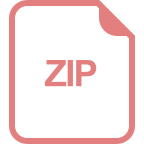
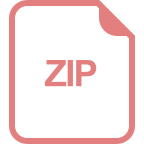
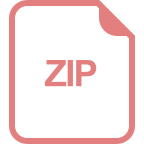
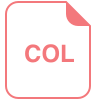
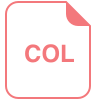
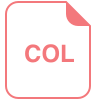
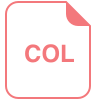










