python二维数组中添加数据
时间: 2023-09-28 22:13:09 浏览: 142
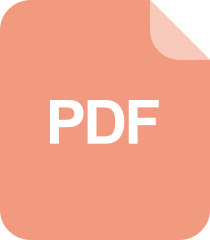
Python中创建二维数组
在 Python 中,二维数组可以使用嵌套列表(即列表中包含列表)来表示。你可以使用 append() 方法向二维数组中添加数据。以下是一个示例:
```python
# 创建一个空的二维数组
my_array = []
# 向二维数组中添加一行数据
row1 = [1, 2, 3]
my_array.append(row1)
# 向二维数组中添加另一行数据
row2 = [4, 5, 6]
my_array.append(row2)
# 打印二维数组
print(my_array)
```
输出:
```
[[1, 2, 3], [4, 5, 6]]
```
在上面的示例中,我们首先创建一个空的二维数组 `my_array`。然后,我们创建两个列表 `row1` 和 `row2`,并将它们分别添加到二维数组中。最后,我们打印二维数组来验证它是否包含了我们添加的数据。
阅读全文
相关推荐
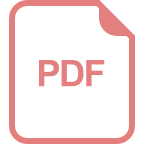













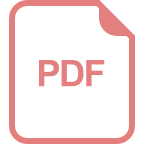
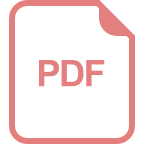
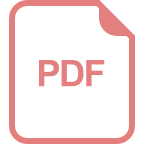

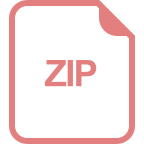