C#实现矩形重叠判定(一个新矩形,多个老矩形,验证新矩形是否与老矩形重叠)
时间: 2024-09-24 20:05:51 浏览: 60
在C#中,要判断一个新矩形是否与一组已知的老矩形重叠,可以使用`Rectangle`类,并利用它们的几何属性进行比较。以下是一个简单的步骤:
1. 定义一个矩形结构或者类`Rectangle`,包含`X`, `Y`, `Width`, `Height`等属性。
```csharp
public class Rectangle
{
public int X { get; set; }
public int Y { get; set; }
public int Width { get; set; }
public int Height { get; set; }
// 构造函数和其他辅助方法
}
```
2. 创建一个方法`IsOverlap(Rectangle newRect, List<Rectangle> oldRectangles)`,其中`newRect`代表新矩形,`oldRectangles`是一个矩形列表,表示所有老矩形。
```csharp
public bool IsOverlap(Rectangle newRect, List<Rectangle> oldRectangles)
{
foreach (Rectangle oldRect in oldRectangles)
{
if (newRect.IntersectsWith(oldRect))
return true; // 如果有任何一个矩形重叠,则返回true
}
return false; // 没有重叠则返回false
}
// IntersectsWith方法用于检查两个矩形是否有交集
bool Intersects(Rectangle rect1, Rectangle rect2) => rect1.Intersection(rect2).HasContent;
Rectangle Intersection(Rectangle rect1, Rectangle rect2) =>
new Rectangle(Math.Max(rect1.X, rect2.X), Math.Max(rect1.Y, rect2.Y),
Math.Min(rect1.Right, rect2.Right) - Math.Max(rect1.X, rect2.X),
Math.Min(rect1.Bottom, rect2.Bottom) - Math.Max(rect1.Y, rect2.Y));
```
3. 调用这个方法,传入新矩形和矩形列表,判断是否重叠。
```csharp
Rectangle newRect = new Rectangle(0, 0, 50, 50); // 新矩形坐标和大小
List<Rectangle> oldRectangles = ...; // 老矩形列表
if (IsOverlap(newRect, oldRectangles))
{
Console.WriteLine("新矩形与老矩形重叠");
}
else
{
Console.WriteLine("新矩形未与老矩形重叠");
}
```
阅读全文
相关推荐
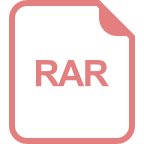
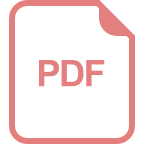
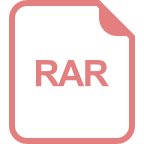

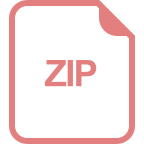
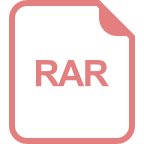
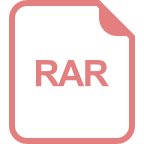
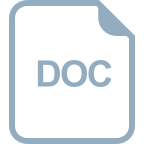
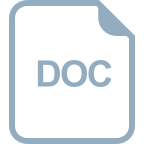
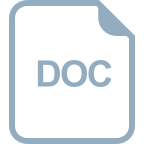








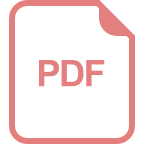