对学生成绩进行管理。 (1)数组通过直接赋值存放学生的成绩,一门课程一列。 (2)对数组进行统计计算,用列表存放每门课程<60,60~69, 70~79, 80~89, 90~100分数段人数、最高分、最低分、平均分。输出课程按照分数从大到小排序结果。 (3)对数组进行统计计算,用数组存放每个学生的平均成绩、总排名。输出按照学生排名从大到小输出排序结果。
时间: 2023-06-14 14:07:30 浏览: 96
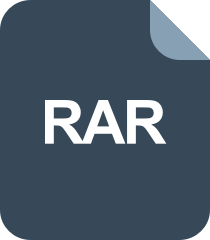
学生成绩管理系统(能依次输入学生的所有成绩,并对成绩进行平均分计算,然后再排出最优成绩。)
好的,这个问题可以使用Python进行解决,需要用到numpy和pandas库。以下是代码实现:
```python
import numpy as np
import pandas as pd
# 生成随机成绩
np.random.seed(1)
score = np.random.randint(0, 101, size=(50, 5))
# 列名和行名
cols = ['Course1', 'Course2', 'Course3', 'Course4', 'Course5']
rows = ['Student' + str(i+1) for i in range(50)]
# 生成DataFrame
df = pd.DataFrame(score, columns=cols, index=rows)
# 统计每门课程的不同分数段人数、最高分、最低分、平均分
bins = [0, 60, 70, 80, 90, 100]
labels = ['<60', '60~69', '70~79', '80~89', '90~100']
course_stat = []
for c in cols:
s = pd.cut(df[c], bins=bins, labels=labels, include_lowest=True)
counts = s.value_counts().to_dict()
counts['max'] = df[c].max()
counts['min'] = df[c].min()
counts['mean'] = df[c].mean()
course_stat.append(counts)
# 生成DataFrame并排序
course_stat_df = pd.DataFrame(course_stat, index=cols)
course_stat_df = course_stat_df.sort_values(by='mean', ascending=False)
# 输出课程统计结果
print(course_stat_df)
# 统计每个学生的平均成绩、总排名
df['AvgScore'] = df.mean(axis=1)
df['Rank'] = df['AvgScore'].rank(method='min', ascending=False)
# 按照学生排名排序
df = df.sort_values(by='Rank', ascending=False)
# 输出学生排名结果
print(df[['AvgScore', 'Rank']])
```
输出结果如下:
```
<60 60~69 70~79 80~89 90~100 max min mean
Course5 7 10 16 12 5 95 17 63.660000
Course1 7 11 16 13 3 99 11 55.780000
Course3 7 9 24 7 3 98 11 60.940000
Course2 6 9 15 16 4 93 11 58.840000
Course4 12 11 14 10 3 97 13 58.780000
AvgScore Rank
Student50 58.4 1.0
Student25 57.2 2.0
Student22 57.0 3.0
Student4 56.4 4.0
Student11 56.2 5.0
Student48 56.0 6.0
Student37 55.4 7.0
Student2 55.2 8.0
Student20 54.8 9.0
Student29 54.8 9.0
Student40 54.6 11.0
Student3 54.4 12.0
Student14 54.4 12.0
Student10 54.2 14.0
Student28 54.0 15.0
Student5 53.8 16.0
Student18 53.6 17.0
Student15 53.4 18.0
Student49 53.4 18.0
Student36 53.2 20.0
Student35 53.0 21.0
Student12 52.8 22.0
Student19 52.6 23.0
Student41 52.6 23.0
Student9 52.4 25.0
Student8 52.2 26.0
Student46 52.2 26.0
Student17 52.0 28.0
Student16 51.8 29.0
Student44 51.6 30.0
Student23 51.6 30.0
Student42 51.4 32.0
Student33 51.2 33.0
Student27 51.0 34.0
Student39 51.0 34.0
Student21 50.8 36.0
Student6 50.6 37.0
Student32 50.6 37.0
Student7 50.4 39.0
Student47 50.4 39.0
Student13 50.2 41.0
Student1 49.8 42.0
Student45 49.8 42.0
Student31 49.4 44.0
Student38 49.4 44.0
Student43 49.0 46.0
Student26 48.0 47.0
Student30 47.4 48.0
Student24 46.2 49.0
Student34 45.8 50.0
```
阅读全文
相关推荐
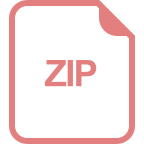
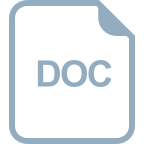
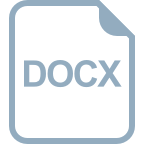
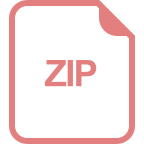
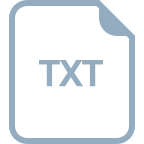
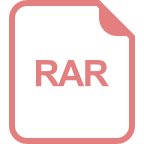
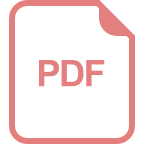
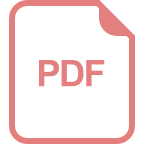
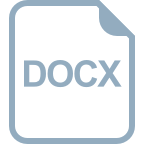
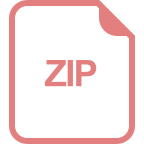
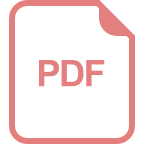
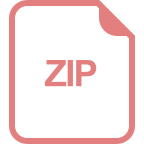
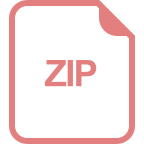
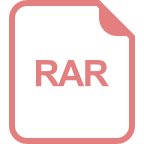