用php写一个购物车功能
时间: 2023-10-08 08:05:11 浏览: 48
好的,以下是一个简单的购物车功能的 PHP 示例代码:
1. 创建一个空的购物车
```
session_start(); // 开启会话
if(!isset($_SESSION['cart'])) {
$_SESSION['cart'] = array();
}
```
2. 添加商品到购物车
```
if(isset($_POST['add_to_cart'])) {
$item_id = $_POST['item_id'];
$item_name = $_POST['item_name'];
$item_price = $_POST['item_price'];
$item_quantity = $_POST['item_quantity'];
$item_total = $item_price * $item_quantity;
$item = array(
'id' => $item_id,
'name' => $item_name,
'price' => $item_price,
'quantity' => $item_quantity,
'total' => $item_total
);
array_push($_SESSION['cart'], $item);
}
```
3. 显示购物车
```
if(isset($_SESSION['cart']) && count($_SESSION['cart']) > 0) {
foreach($_SESSION['cart'] as $item) {
echo "<p>{$item['name']} - 价格: {$item['price']} - 数量: {$item['quantity']} - 总价: {$item['total']}</p>";
}
} else {
echo "购物车为空";
}
```
4. 更新购物车中的商品数量
```
if(isset($_POST['update_cart'])) {
$item_id = $_POST['item_id'];
$item_quantity = $_POST['item_quantity'];
foreach ($_SESSION['cart'] as &$item) {
if ($item['id'] == $item_id) {
$item['quantity'] = $item_quantity;
$item['total'] = $item['price'] * $item_quantity;
break;
}
}
}
```
5. 删除购物车中的商品
```
if(isset($_POST['remove_item'])) {
$item_id = $_POST['item_id'];
foreach ($_SESSION['cart'] as $index => $item) {
if ($item['id'] == $item_id) {
unset($_SESSION['cart'][$index]);
break;
}
}
}
```
注意:以上代码仅供参考,在实际应用中需要考虑到安全性和具体业务逻辑。
相关推荐
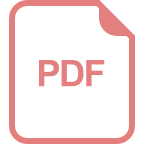
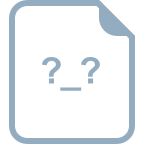
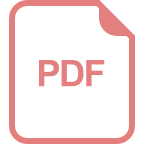
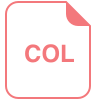













