java 传入多个时间段 返回给定分钟后的日期时间 只考虑工作时段
时间: 2023-12-14 13:35:49 浏览: 67
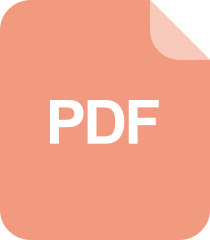
Java默认传入时间段时间的实例
这个问题可以分解为以下几个步骤:
1. 计算出传入的时间段总共有多少分钟。
2. 找到下一个工作日的开始时间。
3. 从下一个工作日的开始时间开始,依次加上每个工作日的工作时间,直到达到传入的分钟数为止。
下面是一个可能的实现:
```java
import java.time.DayOfWeek;
import java.time.LocalDateTime;
import java.time.LocalTime;
import java.time.temporal.ChronoUnit;
import java.util.List;
public class DateTimeUtils {
// 工作日开始时间
private static final LocalTime WORKDAY_START_TIME = LocalTime.of(9, 0);
// 工作日结束时间
private static final LocalTime WORKDAY_END_TIME = LocalTime.of(18, 0);
/**
* 计算给定时间段后的日期时间
* @param startTimes 开始时间列表
* @param endTimes 结束时间列表
* @param minutes 给定分钟数
* @return 计算结果
*/
public static LocalDateTime calculateDateTime(List<LocalDateTime> startTimes, List<LocalDateTime> endTimes, int minutes) {
int totalMinutes = 0;
for (int i = 0; i < startTimes.size(); i++) {
totalMinutes += ChronoUnit.MINUTES.between(startTimes.get(i), endTimes.get(i));
}
LocalDateTime nextWorkdayStart = getNextWorkdayStart(startTimes.get(startTimes.size() - 1).toLocalDate());
LocalDateTime currentDateTime = nextWorkdayStart;
while (totalMinutes < minutes) {
if (currentDateTime.getDayOfWeek() != DayOfWeek.SATURDAY && currentDateTime.getDayOfWeek() != DayOfWeek.SUNDAY) {
LocalTime startTime = WORKDAY_START_TIME;
LocalTime endTime = WORKDAY_END_TIME;
if (currentDateTime.toLocalDate().isEqual(startTimes.get(0).toLocalDate())) {
startTime = startTimes.get(0).toLocalTime();
}
if (currentDateTime.toLocalDate().isEqual(endTimes.get(endTimes.size() - 1).toLocalDate())) {
endTime = endTimes.get(endTimes.size() - 1).toLocalTime();
}
int workMinutes = (int) ChronoUnit.MINUTES.between(startTime, endTime);
totalMinutes += workMinutes;
currentDateTime = currentDateTime.plusMinutes(workMinutes);
} else {
currentDateTime = currentDateTime.plusDays(1);
currentDateTime = LocalDateTime.of(currentDateTime.toLocalDate(), WORKDAY_START_TIME);
}
}
return currentDateTime.minusMinutes(totalMinutes - minutes);
}
/**
* 找到下一个工作日的开始时间
* @param date 当前日期
* @return 下一个工作日的开始时间
*/
private static LocalDateTime getNextWorkdayStart(LocalDateTime currentDateTime) {
LocalDateTime nextWorkdayStart = currentDateTime;
while (nextWorkdayStart.getDayOfWeek() == DayOfWeek.SATURDAY || nextWorkdayStart.getDayOfWeek() == DayOfWeek.SUNDAY) {
nextWorkdayStart = nextWorkdayStart.plusDays(1);
}
return LocalDateTime.of(nextWorkdayStart.toLocalDate(), WORKDAY_START_TIME);
}
}
```
这个实现中,我们首先计算出传入的时间段总共有多少分钟,然后找到下一个工作日的开始时间。接下来,从下一个工作日的开始时间开始,依次加上每个工作日的工作时间,直到达到传入的分钟数为止。如果当前日期是周六或周日,我们就直接跳到下一个工作日的开始时间。最后,我们返回达到给定分钟数时的日期时间。
阅读全文
相关推荐
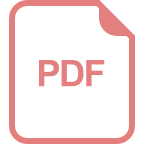
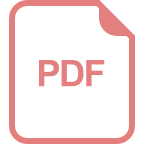















