unity VR轮盘实现车辆的移动与转弯
时间: 2023-12-10 16:42:37 浏览: 94
实现unity VR轮盘控制车辆移动和转弯的步骤如下:
1. 创建一个空物体作为车辆,将车辆模型放置到这个空物体下。
2. 添加一个Rigidbody组件和一个Box Collider组件到车辆上,以实现物理运动和碰撞检测。
3. 创建一个UI Canvas,添加一个Image组件作为轮盘的背景,并将轮盘中心点设置为轮盘的中心位置,再添加一个Image组件作为轮盘指针。
4. 编写代码实现轮盘的旋转和控制车辆的移动和转弯。可以通过监听用户在轮盘上的滑动事件来控制轮盘指针的旋转,然后根据轮盘指针的角度计算车辆应该向前移动的距离和向左或向右转弯的角度。
5. 在Update函数中更新车辆的位置和旋转角度,使其移动和转弯。
下面是一份简单的示例代码,仅供参考:
```c#
using UnityEngine;
using UnityEngine.UI;
public class VRWheelController : MonoBehaviour
{
public float speed = 10f; // 车辆移动速度
public float turnSpeed = 50f; // 车辆转弯速度
public Image wheelImage; // 轮盘指针
private float wheelAngle; // 轮盘指针角度
private Rigidbody rb;
void Start()
{
rb = GetComponent<Rigidbody>();
}
void Update()
{
// 计算车辆应该移动的距离和转弯的角度
float moveDistance = Time.deltaTime * speed;
float turnAngle = Time.deltaTime * turnSpeed;
// 根据轮盘指针的角度计算车辆应该移动的方向和转弯的方向
Vector3 moveDirection = Quaternion.Euler(0f, wheelAngle, 0f) * Vector3.forward;
Quaternion turnDirection = Quaternion.Euler(0f, wheelAngle, 0f);
// 更新车辆的位置和旋转角度
rb.MovePosition(transform.position + moveDirection * moveDistance);
rb.MoveRotation(rb.rotation * turnDirection);
}
// 监听轮盘指针的滑动事件
public void OnPointerDrag()
{
Vector2 pointerPosition = Vector2.zero;
RectTransformUtility.ScreenPointToLocalPointInRectangle(wheelImage.rectTransform, Input.mousePosition, null, out pointerPosition);
// 计算轮盘指针相对于轮盘中心点的角度
wheelAngle = Mathf.Atan2(pointerPosition.y, pointerPosition.x) * Mathf.Rad2Deg;
}
}
```
阅读全文
相关推荐
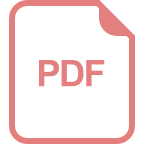
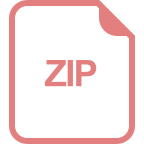
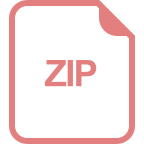
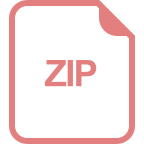
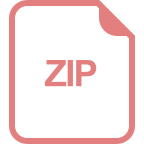
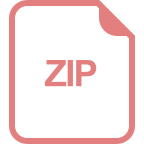
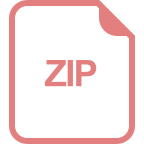
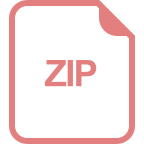
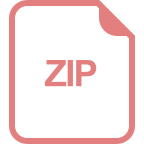
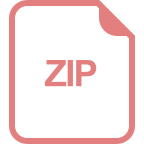
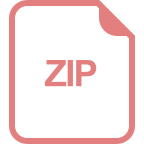
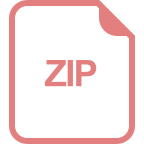
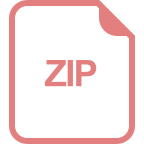
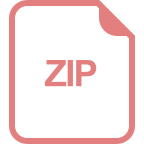
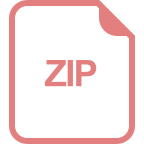