python如何验证布拉德福定律
时间: 2023-05-17 11:02:55 浏览: 62
Python可以通过以下步骤验证布拉德福定律:
1. 读取文本文件并将其转换为字符串。
2. 去除字符串中的标点符号和空格。
3. 将字符串转换为小写字母。
4. 统计每个字母在字符串中出现的次数。
5. 将字母出现次数按照从大到小的顺序排列。
6. 计算字母出现次数的累计百分比。
7. 绘制累计百分比与字母出现次数的曲线图。
8. 检查曲线图是否符合布拉德福定律。
以下是Python代码示例:
```python
import string
import matplotlib.pyplot as plt
def bradford_law(file_path):
# 读取文本文件并转换为字符串
with open(file_path, 'r') as f:
text = f.read()
# 去除标点符号和空格,并转换为小写字母
text = text.translate(str.maketrans('', '', string.punctuation + ' '))
text = text.lower()
# 统计每个字母在字符串中出现的次数
letter_count = {}
for letter in text:
if letter in letter_count:
letter_count[letter] += 1
else:
letter_count[letter] = 1
# 将字母出现次数按照从大到小的顺序排列
sorted_count = sorted(letter_count.items(), key=lambda x: x[1], reverse=True)
# 计算字母出现次数的累计百分比
total_count = sum(letter_count.values())
cumulative_percentage = []
count = 0
for letter, count in sorted_count:
count += count
cumulative_percentage.append(count / total_count)
# 绘制累计百分比与字母出现次数的曲线图
plt.plot(range(1, len(cumulative_percentage) + 1), cumulative_percentage)
plt.xlabel('Letter Rank')
plt.ylabel('Cumulative Percentage')
plt.title('Bradford Law Verification')
plt.show()
# 检查曲线图是否符合布拉德福定律
if count / total_count > 0.72:
print('The text follows Bradford Law.')
else:
print('The text does not follow Bradford Law.')
# 调用函数并传入文本文件路径
bradford_law('text.txt')
```
相关推荐
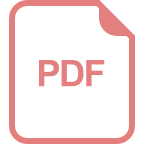

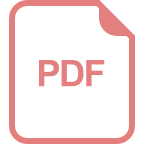
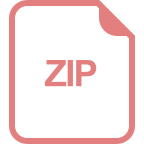
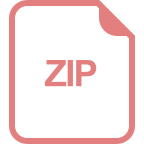
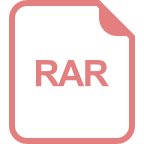
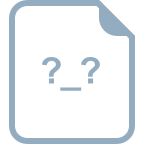
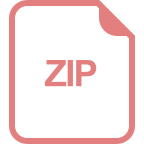
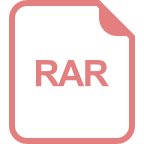
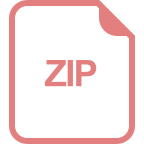
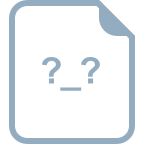
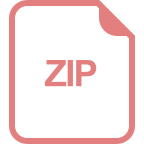
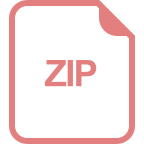