# Task 2: Capture Multiple Groups Write a python program to match the following two regex groups inside a string: To match an UPPERCASE word To match a number Sample data : "The students of SCUT is 30000" Sample output :('SCUT', '30000')
时间: 2024-01-10 12:03:24 浏览: 25
Here's a Python program that uses regular expressions to capture multiple groups from a string:
```python
import re
text = "The students of SCUT is 30000"
pattern = r"([A-Z]+)\s+is\s+(\d+)"
match = re.search(pattern, text)
if match:
print(match.groups())
else:
print("No match")
```
Output:
```
('SCUT', '30000')
```
Explanation:
- The regular expression pattern `([A-Z]+)\s+is\s+(\d+)` consists of two capturing groups enclosed in parentheses.
- The first group `([A-Z]+)` matches one or more uppercase letters.
- The `\s+` matches one or more whitespace characters (spaces, tabs, etc.).
- The literal string `is` matches the word "is".
- The second group `(\d+)` matches one or more digits.
- `re.search(pattern, text)` searches for the pattern in the text and returns a match object if found.
- `match.groups()` returns a tuple of all the captured groups.
相关推荐
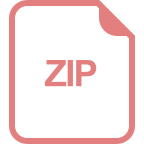
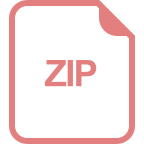
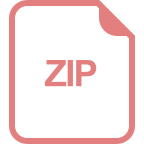














