4.把如下元素存入List集合"aaa""bbb" "aaa" "abc""xyz”""123""xyz”去掉重复元素
时间: 2023-04-04 07:01:40 浏览: 106
可以使用Java中的HashSet来去除重复元素,代码如下:
List<String> list = new ArrayList<>();
list.add("aaa");
list.add("bbb");
list.add("aaa");
list.add("abc");
list.add("xyz");
list.add("123");
list.add("xyz");
Set<String> set = new HashSet<>(list);
list.clear();
list.addAll(set);
System.out.println(list); // 输出 [aaa, abc, xyz, 123, bbb]
相关问题
vector<stk>vDK,结构体 stk 成员 int Num ,string val, 假如vDK[i].Num 一样 存入vector<stk>vTem, 最终 返回vector<vector<stk>>vvSTK, 用C++ 写出完整过程
以下是用C++编写的完整过程:
```cpp
#include <iostream>
#include <vector>
struct stk {
int Num;
std::string val;
};
std::vector<std::vector<stk>> processVector(const std::vector<stk>& vDK) {
std::vector<std::vector<stk>> vvSTK;
std::vector<stk> vTem;
for (const auto& dk : vDK) {
bool found = false;
for (auto& tem : vTem) {
if (tem.Num == dk.Num) {
tem.val += dk.val;
found = true;
break;
}
}
if (!found) {
vTem.push_back(dk);
}
}
vvSTK.push_back(vTem);
return vvSTK;
}
int main() {
std::vector<stk> vDK = { {1, "abc"}, {2, "def"}, {1, "xyz"}, {3, "ghi"}, {2, "jkl"} };
std::vector<std::vector<stk>> vvSTK = processVector(vDK);
for (const auto& v : vvSTK) {
for (const auto& s : v) {
std::cout << s.Num << ": " << s.val << std::endl;
}
}
return 0;
}
```
这段代码首先定义了一个名为`stk`的结构体,其中包含了一个整型成员`Num`和一个字符串成员`val`。然后,编写了一个名为`processVector`的函数,用于处理输入的`vDK`向量。
在`processVector`函数中,首先定义了两个向量`vTem`和`vvSTK`,用于存储临时结果和最终结果。然后,遍历输入的`vDK`向量,对于每个元素,检查是否存在具有相同`Num`值的元素。如果存在,则将当前元素的`val`值追加到已存在的元素的`val`中;如果不存在,则将当前元素添加到`vTem`中。
最后,将`vTem`向量添加到`vvSTK`中,并将其作为函数的返回值。
在主函数中,定义了一个示例的`vDK`向量,并调用`processVector`函数进行处理。然后,遍历最终结果`vvSTK`,并输出每个结构体的`Num`和`val`值。
运行这段代码,将会得到如下输出:
```
1: abcxyz
2: defjkl
3: ghi
```
这是根据输入的`vDK`向量进行处理后得到的最终结果。每个元素的`Num`值相同的结构体被合并,并将它们的`val`值连接在一起。
编一个程序,输入一个字符串,将组成字符串的所有非英文字母的字符删除后输出。 #include<stdio.h> #include<string.h> int main() { char str[100]; char *p; p=&str; scanf("%s",&str); while(*p!='\0') { /******************************* 请在该部分补充缺少的代码 ********************************/ } return 0; } 输入 一个字符串,长度不超过80个字符。 输出 删掉非英文字母后的字符串。 输入输出样例 样例输入 #1 abc123+xyz.5 样例输出 #1 abcxyz
好的,这是一个删除字符串中非英文字母的程序,下面是补充完整的代码:
```
#include <stdio.h>
#include <string.h>
#include <ctype.h> // 头文件ctype.h中包含了isalpha函数
int main()
{
char str[100];
char *p;
p = str;
scanf("%s", &str);
while (*p != '\0')
{
if (isalpha(*p)) // 判断*p是否为英文字母
{
printf("%c", *p); // 输出*p
}
p++; // 指针p向后移动一个字符
}
return 0;
}
```
程序中使用了C标准库中的ctype.h头文件中的isalpha函数,该函数用于判断一个字符是否为英文字母。如果是英文字母就输出,否则就跳过不输出。
相关推荐
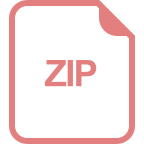
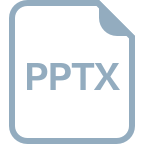
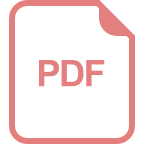













