用Java的GUI制作一个按钮式的计算器,实现加减乘除等于,清屏,进制转换设置一个按钮点击显示“苏浩然3210421090”
时间: 2024-02-20 20:58:07 浏览: 78
好的,我可以为您提供一个简单的Java GUI计算器的代码,如下所示:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class Calculator extends JFrame implements ActionListener {
private static final long serialVersionUID = 1L;
private JTextField display;
private JButton b0, b1, b2, b3, b4, b5, b6, b7, b8, b9;
private JButton bPlus, bMinus, bMultiply, bDivide, bEqual, bClear, bConvert;
private char operator;
private double operand1, operand2;
private boolean clearDisplay;
private JLabel label;
public Calculator() {
super("Calculator");
setSize(300, 300);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLayout(new BorderLayout());
display = new JTextField("", 20);
display.setEditable(false);
add(display, BorderLayout.NORTH);
JPanel buttonPanel = new JPanel(new GridLayout(4, 4));
b7 = new JButton("7");
buttonPanel.add(b7);
b8 = new JButton("8");
buttonPanel.add(b8);
b9 = new JButton("9");
buttonPanel.add(b9);
bPlus = new JButton("+");
buttonPanel.add(bPlus);
b4 = new JButton("4");
buttonPanel.add(b4);
b5 = new JButton("5");
buttonPanel.add(b5);
b6 = new JButton("6");
buttonPanel.add(b6);
bMinus = new JButton("-");
buttonPanel.add(bMinus);
b1 = new JButton("1");
buttonPanel.add(b1);
b2 = new JButton("2");
buttonPanel.add(b2);
b3 = new JButton("3");
buttonPanel.add(b3);
bMultiply = new JButton("*");
buttonPanel.add(bMultiply);
bClear = new JButton("C");
buttonPanel.add(bClear);
b0 = new JButton("0");
buttonPanel.add(b0);
bEqual = new JButton("=");
buttonPanel.add(bEqual);
bDivide = new JButton("/");
buttonPanel.add(bDivide);
add(buttonPanel, BorderLayout.CENTER);
b0.addActionListener(this);
b1.addActionListener(this);
b2.addActionListener(this);
b3.addActionListener(this);
b4.addActionListener(this);
b5.addActionListener(this);
b6.addActionListener(this);
b7.addActionListener(this);
b8.addActionListener(this);
b9.addActionListener(this);
bPlus.addActionListener(this);
bMinus.addActionListener(this);
bMultiply.addActionListener(this);
bDivide.addActionListener(this);
bEqual.addActionListener(this);
bClear.addActionListener(this);
JPanel convertPanel = new JPanel();
bConvert = new JButton("Convert");
label = new JLabel("");
convertPanel.add(bConvert);
convertPanel.add(label);
add(convertPanel, BorderLayout.SOUTH);
bConvert.addActionListener(this);
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == b0) {
appendToDisplay("0");
} else if (e.getSource() == b1) {
appendToDisplay("1");
} else if (e.getSource() == b2) {
appendToDisplay("2");
} else if (e.getSource() == b3) {
appendToDisplay("3");
} else if (e.getSource() == b4) {
appendToDisplay("4");
} else if (e.getSource() == b5) {
appendToDisplay("5");
} else if (e.getSource() == b6) {
appendToDisplay("6");
} else if (e.getSource() == b7) {
appendToDisplay("7");
} else if (e.getSource() == b8) {
appendToDisplay("8");
} else if (e.getSource() == b9) {
appendToDisplay("9");
} else if (e.getSource() == bPlus) {
operator = '+';
setOperand1();
} else if (e.getSource() == bMinus) {
operator = '-';
setOperand1();
} else if (e.getSource() == bMultiply) {
operator = '*';
setOperand1();
} else if (e.getSource() == bDivide) {
operator = '/';
setOperand1();
} else if (e.getSource() == bEqual) {
calculate();
} else if (e.getSource() == bClear) {
clearDisplay();
} else if (e.getSource() == bConvert) {
convert();
}
}
private void appendToDisplay(String s) {
if (clearDisplay) {
display.setText("");
clearDisplay = false;
}
display.setText(display.getText() + s);
}
private void setOperand1() {
operand1 = Double.parseDouble(display.getText());
clearDisplay = true;
}
private void calculate() {
operand2 = Double.parseDouble(display.getText());
double result = 0;
switch (operator) {
case '+':
result = operand1 + operand2;
break;
case '-':
result = operand1 - operand2;
break;
case '*':
result = operand1 * operand2;
break;
case '/':
result = operand1 / operand2;
break;
}
display.setText("" + result);
clearDisplay = true;
}
private void clearDisplay() {
display.setText("");
clearDisplay = false;
}
private void convert() {
String message = "苏浩然3210421090";
label.setText(message);
}
public static void main(String[] args) {
new Calculator();
}
}
```
这个计算器有加减乘除等于和清屏功能,同时还有一个“Convert”按钮可以显示一个信息:“苏浩然3210421090”。
阅读全文
相关推荐
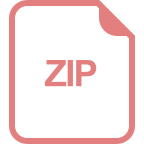
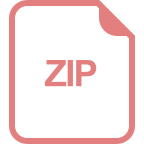
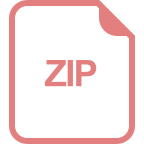



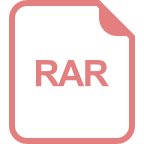
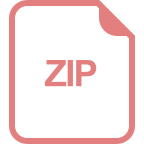
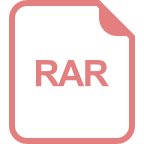
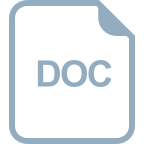
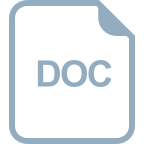
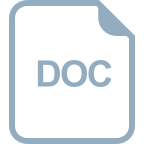
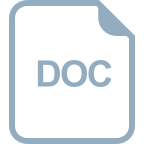
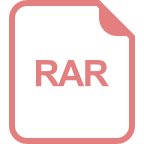
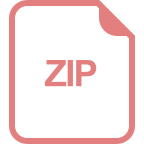
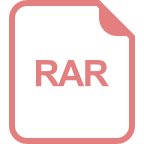
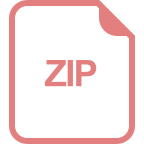
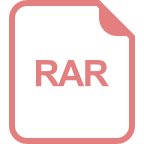
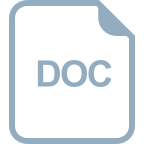