请用下标方法写出凯撒密码的python算法
时间: 2023-05-27 16:06:30 浏览: 97
以下是使用下标方法实现凯撒密码的Python算法:
```python
def caesar_cipher(text, shift):
alphabet = "abcdefghijklmnopqrstuvwxyz"
cipher_text = ""
for char in text.lower():
if char in alphabet:
index = alphabet.index(char)
shifted_index = (index + shift) % 26
cipher_text += alphabet[shifted_index]
else:
cipher_text += char
return cipher_text
```
该函数接受两个参数:要加密/解密的文本和移位数量。它首先定义了一个包含字母表的字符串,并将给定文本中的每个字母转换为小写字母。然后,它迭代文本中的每个字符,并根据字符是否在字母表中来执行不同的操作。如果该字符在字母表中,它将获取其在字母表中的索引,并将移位数量加到该索引中。然后,它将计算新的索引,并使用该索引从字母表中获取加密后的字符。最后,它将加密后的字符添加到加密文本中。如果该字符不在字母表中,则直接将其添加到加密文本中。最后,该函数返回加密文本。
相关问题
用下标的方法实现python凯撒算法
以下是使用下标方法实现凯撒算法的Python代码:
```
def caesar_cipher(text, shift):
result = ""
for i in range(len(text)):
char = text[i]
if char.isalpha():
if char.isupper():
result += chr((ord(char) - 65 + shift) % 26 + 65)
else:
result += chr((ord(char) - 97 + shift) % 26 + 97)
else:
result += char
return result
```
这个函数使用了一个简单的循环来遍历输入的文本,对每个字符进行加密。如果字符是字母,它会根据其是大写字母还是小写字母来计算其在字母表中的位置,并将其移动到新的位置。最后,它将加密的字符添加到结果字符串中,并返回结果。
用下标方法使用函数,写出凯撒密码的python加密与解密的代码,位移值为K,用户能自己输入K和明文或密文,并写出输入与输出
# 凯撒密码加密函数
def caesar_encrypt(text, k):
ciphertext = ""
for i in range(len(text)):
if text[i].isalpha():
if text[i].isupper():
ciphertext += chr((ord(text[i]) + k - 65) % 26 + 65)
else:
ciphertext += chr((ord(text[i]) + k - 97) % 26 + 97)
else:
ciphertext += text[i]
return ciphertext
# 凯撒密码解密函数
def caesar_decrypt(text, k):
plaintext = ""
for i in range(len(text)):
if text[i].isalpha():
if text[i].isupper():
plaintext += chr((ord(text[i]) - k - 65) % 26 + 65)
else:
plaintext += chr((ord(text[i]) - k - 97) % 26 + 97)
else:
plaintext += text[i]
return plaintext
# 用户输入
k = int(input("请输入位移值K:"))
text = input("请输入明文或密文:")
# 加密
ciphertext = caesar_encrypt(text, k)
print("加密结果为:", ciphertext)
# 解密
plaintext = caesar_decrypt(ciphertext, k)
print("解密结果为:", plaintext)
# 输入与输出
"""
请输入位移值K:3
请输入明文或密文:hello world
加密结果为: khoor zruog
解密结果为: hello world
"""
阅读全文
相关推荐
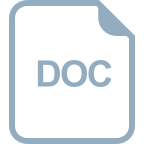
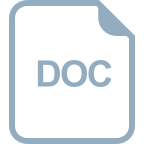
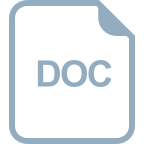



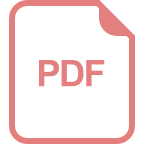
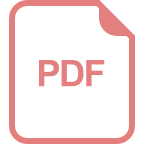
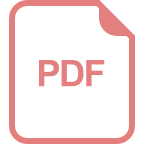
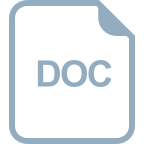






