C# fstream
时间: 2023-10-19 17:02:25 浏览: 45
C#中的`FileStream`是用于读取和写入二进制数据的类。它提供了访问文件的底层功能,可以在文件中进行读写操作。
为了使用`FileStream`类,你需要在代码中引入`System.IO`命名空间。下面是一个简单的示例,展示了如何使用`FileStream`来打开一个文件并进行读取操作:
```csharp
using System;
using System.IO;
class Program
{
static void Main()
{
// 文件路径
string filePath = "example.txt";
// 使用FileStream打开文件
using (FileStream fs = new FileStream(filePath, FileMode.Open))
{
// 创建一个字节数组用于存储读取的数据
byte[] buffer = new byte
相关问题
c#解析protobuf
要在 C++ 中解析 protobuf,你需要先安装 protobuf 库并将其添加到你的项目中。然后,你需要使用 protobuf 编译器将 .proto 文件编译成 C++ 文件。接着,你可以使用 protobuf 库提供的 API 来解析 protobuf 数据。
下面是一个简单的示例代码:
```c++
#include <iostream>
#include <fstream>
#include "my_proto.pb.h" // 编译器生成的头文件
using namespace std;
int main() {
// 从文件中读取 protobuf 数据
fstream input("my_proto.bin", ios::in | ios::binary);
MyProto my_proto;
if (!my_proto.ParseFromIstream(&input)) {
cerr << "Failed to parse MyProto." << endl;
return -1;
}
// 访问 protobuf 数据中的字段
cout << "Field1: " << my_proto.field1() << endl;
cout << "Field2: " << my_proto.field2() << endl;
return 0;
}
```
在这个示例中,我们首先使用 `fstream` 从文件中读取 protobuf 数据。然后,我们使用 `MyProto` 类提供的访问器函数来访问 protobuf 数据中的字段。在这个示例中,我们访问了 `field1` 和 `field2` 两个字段。
注意,这里的 `MyProto` 类是由编译器生成的,它包含了 `.proto` 文件中定义的消息类型和访问器函数。如果你修改了 `.proto` 文件,你需要重新编译生成新的 `MyProto` 类。
c++ fstream有flush吗
在 C++ 中,`std::fstream` 类也有 `flush()` 方法,它和 C# 中的 `Stream.Flush()` 方法功能类似。调用 `flush()` 方法可以将缓冲区中的数据强制刷新到文件中,确保数据被写入磁盘而不是仅仅存储在缓冲区中。
`std::fstream` 类中的 `flush()` 方法的具体用法和 C# 中的 `Stream.Flush()` 方法相同,你可以在读写文件时使用它来确保数据被正确地写入文件。例如,你可以在写入文件后调用 `flush()` 方法来确保数据被写入磁盘:
```cpp
#include <fstream>
int main() {
std::fstream file("example.txt", std::ios::out);
if (file.is_open()) {
file << "Hello, world!";
file.flush(); // 将缓冲区中的数据写入文件
file.close();
}
return 0;
}
```
需要注意的是,`flush()` 方法并不会关闭文件,如果需要关闭文件,还需要调用 `close()` 方法。
相关推荐
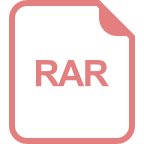
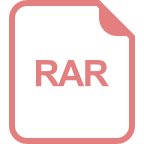
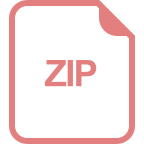










