用c sharp 生成 五子棋小游戏
时间: 2023-05-13 22:06:05 浏览: 81
你可以使用 C# 编写五子棋小游戏。你需要创建一个棋盘,然后在棋盘上放置棋子。你可以使用 Windows Forms 或 WPF 来创建用户界面。你还需要编写一些逻辑来判断胜利条件和防止玩家作弊。以下是一个简单的示例代码:
```csharp
using System;
using System.Windows.Forms;
namespace Gomoku
{
public partial class MainForm : Form
{
private const int BoardSize = 15;
private const int CellSize = 30;
private const int Margin = 50;
private bool[,] board = new bool[BoardSize, BoardSize];
private bool isBlackTurn = true;
public MainForm()
{
InitializeComponent();
this.ClientSize = new System.Drawing.Size(BoardSize * CellSize + Margin * 2, BoardSize * CellSize + Margin * 2);
this.Paint += MainForm_Paint;
this.MouseClick += MainForm_MouseClick;
}
private void MainForm_Paint(object sender, PaintEventArgs e)
{
for (int i = 0; i < BoardSize; i++)
{
for (int j = 0; j < BoardSize; j++)
{
e.Graphics.DrawRectangle(Pens.Black, Margin + i * CellSize, Margin + j * CellSize, CellSize, CellSize);
if (board[i, j])
{
e.Graphics.FillEllipse(isBlackTurn ? Brushes.Black : Brushes.White, Margin + i * CellSize, Margin + j * CellSize, CellSize, CellSize);
}
}
}
}
private void MainForm_MouseClick(object sender, MouseEventArgs e)
{
int x = (e.X - Margin) / CellSize;
int y = (e.Y - Margin) / CellSize;
if (x >= 0 && x < BoardSize && y >= 0 && y < BoardSize && !board[x, y])
{
board[x, y] = true;
isBlackTurn = !isBlackTurn;
this.Invalidate();
}
}
}
}
```
这个代码使用 Windows Forms 创建了一个窗口,然后在窗口上绘制了一个棋盘。当玩家点击棋盘时,程序会在对应的位置放置棋子,并切换到另一个玩家的回合。
相关推荐
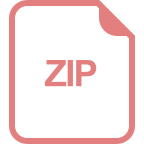
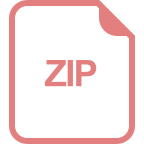
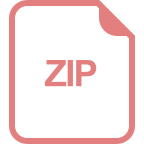


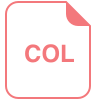
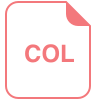
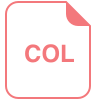









