Springboot 实现curl调用
时间: 2024-01-28 14:03:48 浏览: 47
要在Spring Boot中实现Curl调用,您可以使用Java的HttpURLConnection或Apache HttpClient库。下面是使用Apache HttpClient库的示例代码:
首先,确保在您的项目中包含以下Maven依赖项:
```xml
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.13</version>
</dependency>
```
然后,您可以创建一个CurlUtil类来执行Curl调用:
```java
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
import java.io.IOException;
public class CurlUtil {
public static String sendGetRequest(String url) throws IOException {
CloseableHttpClient httpClient = HttpClients.createDefault();
HttpGet httpGet = new HttpGet(url);
HttpResponse response = httpClient.execute(httpGet);
HttpEntity entity = response.getEntity();
String responseString = EntityUtils.toString(entity);
httpClient.close();
return responseString;
}
}
```
现在,您可以在您的Spring Boot应用程序中使用CurlUtil类来进行Curl调用。例如,您可以在控制器中使用以下代码:
```java
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/api")
public class MyController {
@GetMapping("/curl")
public String performCurlCall() {
try {
String response = CurlUtil.sendGetRequest("YOUR_CURL_URL");
return response;
} catch (IOException e) {
e.printStackTrace();
return "Error occurred while performing Curl call.";
}
}
}
```
请将 "YOUR_CURL_URL" 替换为实际的Curl URL。现在,当您访问`http://localhost:8080/api/curl`时,将执行Curl调用并返回响应结果。
这是使用Spring Boot实现Curl调用的基本示例。根据您的需求,您可以扩展此示例以满足更复杂的需求。
相关推荐












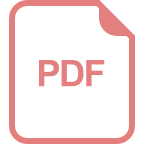
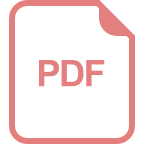