用python写一个雪花飘落的程序
时间: 2023-03-19 16:24:45 浏览: 172
下面是一个使用 Python 的 Pygame 库实现的简单的雪花飘落程序:
```python
import pygame
import random
# 初始化 Pygame 库
pygame.init()
# 定义屏幕大小和帧率
screen_width = 640
screen_height = 480
fps = 30
# 创建游戏窗口
screen = pygame.display.set_mode((screen_width, screen_height))
# 设置游戏窗口标题
pygame.display.set_caption("Snowflake Simulation")
# 定义雪花对象
class Snowflake:
def __init__(self):
self.x = random.randint(0, screen_width)
self.y = -10
self.speed = random.randint(1, 5)
self.size = random.randint(5, 10)
def update(self):
self.y += self.speed
if self.y > screen_height:
self.y = -10
self.x = random.randint(0, screen_width)
def draw(self):
pygame.draw.circle(screen, (255, 255, 255), (self.x, self.y), self.size)
# 创建雪花列表
snowflakes = []
for i in range(100):
snowflakes.append(Snowflake())
# 创建游戏时钟对象
clock = pygame.time.Clock()
# 游戏循环
running = True
while running:
# 处理游戏事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 更新雪花位置
for snowflake in snowflakes:
snowflake.update()
# 绘制游戏画面
screen.fill((0, 0, 0))
for snowflake in snowflakes:
snowflake.draw()
pygame.display.flip()
# 控制游戏帧率
clock.tick(fps)
# 退出游戏
pygame.quit()
```
运行该程序后,会在窗口中看到雪花飘落的效果。可以根据需要调整屏幕大小、帧率、雪花数量、速度、大小等参数来修改程序。
相关推荐
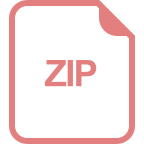














