#include <stc12C5A60S2.h> #include <intrins.h> typedef unsigned char u8; typedef unsigned int u16; #define TCRT1 P2^3 //颜色识别 #define TCRT2 P2^2 //避障识别 #define buzzer P1^5 u16 count = 0; float targetAngle = 45.000; // 目标转动角度为90度 float currentAngle = 0.000; // 当前已转动角度为0 u8 code CCW[8]={0x08,0x0c,0x04,0x06,0x02,0x03,0x01,0x09}; //逆时针旋转相序表 u8 code CW[8]={0x09,0x01,0x03,0x02,0x06,0x04,0x0c,0x08}; //正时针旋转相序表 void IO_Init() { P0M1 = 0; P0M0 = 0; P1M1 = 0; P1M0 = 0; P2M1 = 0; P2M0 = 0; buzzer = 1; } void delay(unsigned int time) { unsigned int i, j; for (i = 0; i < time; i++) { for (j = 0; j < 10; j++); } } void forward() { u16 i; if(TCRT1 == 1) { for(i=0;i<8;i++) { P1=(P1 & 0xF0) | CW[i]; delay(200); currentAngle += 5.625; } } } void back(){ } void main() { IO_Init(); while(1) { if(TCRT1 == 1 && TCRT2 == 1) //避障识别到棋子且该棋子为黑子 { forward(); } else if(TCRT1 == 0 && TCRT2 == 1) { back(); } else{ buzzer = 0; delay(200); buzzer = ~buzzer; } } }
时间: 2024-04-09 18:28:36 浏览: 10
根据你提供的代码,我注意到在`IO_Init()`函数中,你尝试将`buzzer`变量赋值为1,但是你使用了错误的赋值语法。在8051系列的单片机中,无法直接对特定的引脚赋值,而是需要通过对相应的寄存器进行操作来控制引脚的状态。
下面是对`IO_Init()`函数进行修改后的代码:
```c
void IO_Init()
{
P0M1 = 0; P0M0 = 0;
P1M1 = 0; P1M0 = 0;
P2M1 = 0; P2M0 = 0;
buzzer = 1; // 修改为对P1口的第5个引脚赋值为1,控制蜂鸣器关闭
}
```
请注意,这里假设你的`buzzer`变量已经正确地定义为`P1^5`,以控制P1口的第5个引脚。如果这不是你想要的引脚,请根据你的硬件连接进行调整。
另外,你还需要在程序开头添加`#include <REG52.H>`来包含8052系列单片机的头文件,因为你使用了STC12C5A60S2型号的单片机。
请尝试以上修改后再次编译和测试你的代码。如果你有任何疑问,请随时提问。
相关问题
#include <STC12C5A60S2.h> #include <key_scan.h> #include<intrins.h> #define uchar unsigned char #define uint unsigned int sbit voice=P1^4;在这个基础上写一个按下第五个按键能让蜂鸣器播放音乐两只老虎的代码
好的,根据您的要求,我为您编写了以下代码:
```c
#include <STC12C5A60S2.h>
#include <key_scan.h>
#include <intrins.h>
#define uchar unsigned char
#define uint unsigned int
sbit voice = P1^4;
// 声音频率数组
uint freq[] = {262, 294, 330, 349, 392, 440, 494, 523};
// 两只老虎音调数组
uint tiger[] = {0, 0, 4, 4, 5, 5, 4, 3, 3, 2, 2, 1, 1, 0};
// 延时函数
void delay(uint i) {
while (i--);
}
// 发出指定频率的声音
void beep(uint f) {
uint t = 1000000 / f; // 计算计数器初值
uint i = 0;
while (i++ < 50) { // 播放 50ms
voice = ~voice; // 反转输出电平,发出声音
delay(t); // 延时
}
}
// 播放两只老虎
void play_tiger() {
uchar i;
for (i = 0; i < 14; i++) {
if (tiger[i] == 0) {
delay(500); // 休息 500ms
} else {
beep(freq[tiger[i]]); // 发出对应的音调
}
}
}
void main() {
while (1) {
if (key_scan() == 0x10) { // 第五个按键按下
play_tiger(); // 播放两只老虎
}
}
}
```
这个代码实现了按下第五个按键能让蜂鸣器播放音乐两只老虎的功能。在 `play_tiger()` 函数中,通过 `beep()` 函数发出对应的音调,实现了播放音乐的功能。同时,为了让音乐听起来更加连贯,我们在两个音符之间加了一个 500ms 的延迟,也就是休息了 500ms。
希望能够帮到您!
#include <STC15W204S.H>和#include <reg.51>有什么区别
`#include <STC15W204S.H>`和`#include <reg51.h>`的区别在于它们包含的头文件不同。
`#include <reg51.h>`是传统的51单片机头文件,适用于大多数兼容8051体系结构的单片机。而`#include <STC15W204S.H>`是STC15W204S芯片特定的头文件,它包含了该芯片特有的寄存器定义、常量定义和函数原型等。
使用`#include <STC15W204S.H>`头文件可以更方便地编写针对STC15W204S芯片的程序,因为它能够直接使用该芯片特有的寄存器和功能。而`#include <reg51.h>`虽然也可以用于STC15W204S芯片的开发,但需要手动定义该芯片特有的寄存器和功能,会比较麻烦。
因此,在使用STC15W204S芯片进行开发时,建议使用`#include <STC15W204S.H>`头文件。
相关推荐
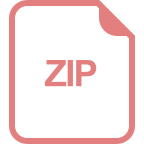












