golang的fyne库实列
时间: 2023-09-10 14:09:59 浏览: 140
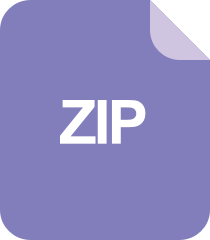
golang GUI图形界面框架fyne演示例子
下面是一个使用 Go 语言的 Fyne 库创建一个简单窗口和按钮的示例:
```go
package main
import (
"fyne.io/fyne/v2"
"fyne.io/fyne/v2/app"
"fyne.io/fyne/v2/widget"
)
func main() {
// 创建一个应用程序实例
myApp := app.New()
// 创建一个窗口
myWindow := myApp.NewWindow("Fyne Example")
// 创建一个按钮
myButton := widget.NewButton("Click me!", func() {
// 按钮点击事件处理
println("Button clicked!")
})
// 将按钮添加到窗口中
myWindow.SetContent(myButton)
// 显示窗口并运行应用程序
myWindow.ShowAndRun()
}
```
这个示例演示了如何使用 Fyne 库创建一个简单的窗口,并在窗口中添加一个按钮。当按钮被点击时,会在控制台输出一条消息。
确保你已经正确安装了 Fyne 库,可以使用以下命令进行安装:
```
go get fyne.io/fyne/v2
```
运行以上代码,你将看到一个包含一个按钮的窗口,点击按钮时会在控制台输出消息。
希望这个示例能够帮助你入门 Fyne 库的使用。如果还有其他问题,请随时提问。
阅读全文
相关推荐
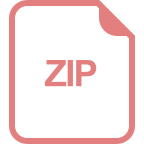

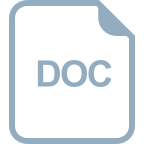
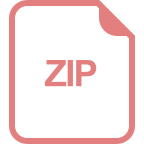
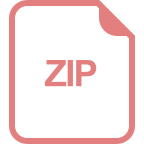
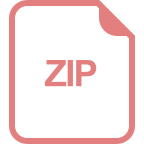
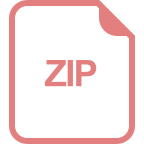
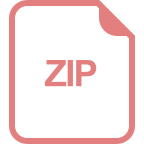
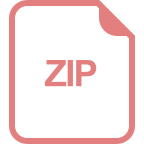
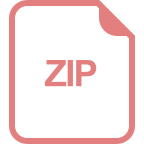
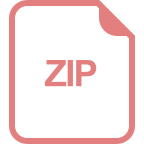
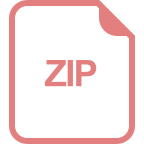
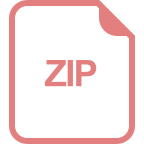
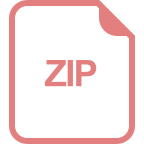
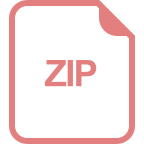
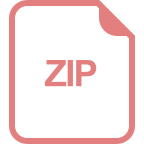