编写一个程序,由函数main和函数fun组成。函数fun(chars, int t)功能是:统计形参s所指字符串中''字符出现的次数,并存放在形参t所指的变量中,最后在主函数中输出
时间: 2024-05-08 10:19:48 浏览: 70
以下是C++语言的代码实现:
```C++
#include <iostream>
#include <cstring>
using namespace std;
void fun(char *chars, int *t) {
int count = 0;
for (int i = 0; i < strlen(chars); i++) {
if (chars[i] == '\'') {
count++;
}
}
*t = count; // 将计数器赋值给t所指的变量
}
int main() {
char str[] = "Hello, 'world'! How 'are' you?";
int count = 0;
fun(str, &count); // 调用函数fun,并将count的地址传递给形参t
cout << "The number of ' in the string is: " << count << endl;
return 0;
}
```
输出结果:
```
The number of ' in the string is: 4
```
说明:以上代码中,函数fun的形参使用指针类型,可以在函数内部修改实参的值,即将计数器count的值赋给形参t所指向的变量。在主函数中,将字符串和计数器count作为实参传递给函数fun,并输出统计结果。
相关问题
用C++ 虛基类 声明一个人员类(Person): 保护数据成员:string name; //姓名 char sex; //性别 int age; //年龄 公有成员西数:Teacher(string n, char s, inta):/构造函数 声明一个教师类(Teacher):公有继承人员类(Person),井声明Person为虚基类保护数据成员:string title; //职称 公有成员函数:Teacher(string n, char s, int a, string t);1构造函数 声明一个学生类(Student):公有继承人员类(Person),并声明Person为虚基类保护数据成员:1oat score;1/成绩 公有成员函数:Student(string n, chars, int a, nloat c):/构造函数 声明一个在职研究生(Graduate), 公有继承教师类(Teacher)和学生类(Student 私有数据成员:noat wage; //工资 公有成员西数:Graduate (string n, char s, int a, string t, float c, float w):/构造西数void showO;1/输出研究生的有关数据(姓名、性别、年龄、职称、成绩、工资) 编写一个程序测试该类,测试数据及要求如下: 主函数中定义在职研究生类对象:Graduate g('LiMing',’f,24,“助教:,89.5,3000); 本程序的执行结果如下: name: LiMing sex: f age:24 title:助教 score: 89.5 wage: 3000
以下是代码实现:
```c++
#include <iostream>
#include <string>
using namespace std;
class Person {
protected:
string name;
char sex;
int age;
public:
Person(string n, char s, int a): name(n), sex(s), age(a) {}
};
class Teacher : virtual public Person {
protected:
string title;
public:
Teacher(string n, char s, int a, string t): Person(n, s, a), title(t) {}
};
class Student : virtual public Person {
protected:
float score;
public:
Student(string n, char s, int a, float c): Person(n, s, a), score(c) {}
};
class Graduate : public Teacher, public Student {
private:
float wage;
public:
Graduate(string n, char s, int a, string t, float c, float w): Person(n, s, a), Teacher(n, s, a, t), Student(n, s, a, c), wage(w) {}
void show() {
cout << "name: " << name << endl;
cout << "sex: " << sex << endl;
cout << "age: " << age << endl;
cout << "title: " << title << endl;
cout << "score: " << score << endl;
cout << "wage: " << wage << endl;
}
};
int main() {
Graduate g("LiMing", 'f', 24, "助教", 89.5, 3000);
g.show();
return 0;
}
```
执行结果为:
```
name: LiMing
sex: f
age: 24
title: 助教
score: 89.5
wage: 3000
```
基于#include <stdio.h> int fcount(char str[],char s); int main(void) { char str[80],s; gets(str); s=getchar(); printf("%d",fcount(str,s)); return 0; } /*考生在以下空白处定义函数*/ /*考生在以上空白处定义函数*/输入一个字符串,统计字符串中指定字符出现次数并输出。【输入格式】 在第一行中输入不超过80个字符长度的、以回车结束的非空字符串:在第二行中输入1个指定字符。【输出格式】 在一行中输出字符串中指定字符的出现次数。【输入样例】 Hello World!1 【输出样例】3 【函数定义要求 请在该程序文件中,实现函数int fcount(charstr1.chars)统计字符串str中字符s的出现次数,并作为函数的返回结果。
好的,这是相应的代码:
```c
#include <stdio.h>
int fcount(char str[], char s) {
int count = 0;
for (int i = 0; str[i] != '\0'; i++) {
if (str[i] == s) {
count++;
}
}
return count;
}
int main(void) {
char str[80], s;
gets(str);
s = getchar();
printf("%d", fcount(str, s));
return 0;
}
```
在这个程序中,我们首先定义了一个函数 `fcount`,它有两个参数,分别是指向字符数组的指针 `str` 和字符 `s`。函数的作用是统计字符串 `str` 中指定字符 `s` 出现的次数,并返回这个次数。
在主程序中,我们首先定义了一个字符数组 `str` 和一个字符变量 `s`。然后从标准输入中读入一个字符串 `str` 和一个字符 `s`。接着调用 `fcount` 函数,将统计结果打印出来。
注意,在 `gets` 函数中,我们没有设定最大字符数,因为题目规定字符串长度不超过 80。另外,在 `fcount` 函数中,我们使用了 C 语言中的字符串结束符 `'\0'` 来判断字符串是否结束。
阅读全文
相关推荐
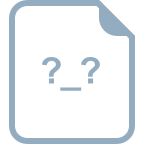
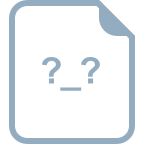










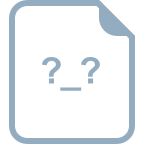