在liunx环境下,编写Python3代码,处理统计/opt/programs/myprog/work.txt文件中单词次数
时间: 2023-12-26 12:07:16 浏览: 65
好的,您可以使用Python的标准库中的collections模块中的Counter类来实现。下面是一个简单的示例代码:
```python
from collections import Counter
# 打开文件,读取内容
with open('/opt/programs/myprog/work.txt', 'r') as f:
words = f.read().split()
# 统计单词出现次数
word_counts = Counter(words)
# 输出结果
for word, count in word_counts.items():
print(f'{word}: {count}')
```
这里首先使用Python的with语句打开文件并读取内容,然后使用split()方法将内容按照空格分隔成单词列表。接着使用Counter类统计单词出现次数,并遍历输出结果。
相关问题
在liunx中,我有如下命令: /home/infa/Grafana/prometheus-2.45.0/promtool check config /home/infa/python/PythonFile/测试文件夹/ymlTest.yml 请写一个python3程序执行该命令
你可以使用`subprocess`模块在Python中执行命令。以下是一个示例代码,用于执行给定的命令:
```python
import subprocess
command = "/home/infa/Grafana/prometheus-2.45.0/promtool check config /home/infa/python/PythonFile/测试文件夹/ymlTest.yml"
try:
output = subprocess.check_output(command, shell=True, stderr=subprocess.STDOUT)
print(output.decode('utf-8'))
except subprocess.CalledProcessError as e:
print(f"Command execution failed with return code {e.returncode}:")
print(e.output.decode('utf-8'))
```
这段代码使用`subprocess.check_output()`函数执行给定的命令,并捕获标准输出。如果命令执行成功,它会打印输出结果;如果命令执行失败,它会打印返回码和错误输出。
请注意,`subprocess.check_output()`函数在命令执行失败时会引发`subprocess.CalledProcessError`异常,因此我们使用了try-except块来处理异常情况。
确保替换`command`变量为你所需的完整命令,然后运行此Python脚本,它将执行该命令并输出结果。
在liunx中如何将python代码打包成可执行文件
可以使用pyinstaller将Python代码打包成可执行文件。下面是一些步骤:
1. 安装pyinstaller:可以使用pip install pyinstaller命令进行安装。
2. 进入到你的Python代码所在的目录。
3. 执行以下命令:pyinstaller your_script.py
4. pyinstaller将自动查找依赖项并将它们打包到可执行文件中。
5. 打包完成后,在dist目录下会生成可执行文件。
注意:在打包过程中,如果你的Python代码中使用了第三方库,则需要先使用pip安装这些库。并且,有些第三方库可能需要手动指定pyinstaller的参数才能正确打包,可以去pyinstaller官网查看相关文档。
阅读全文
相关推荐
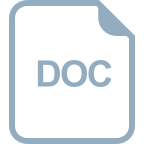
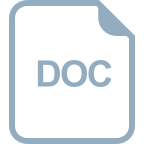
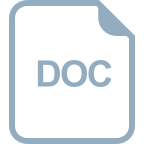
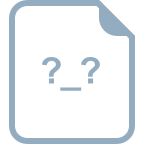
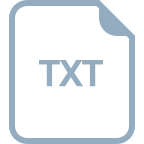






