python-opencv轮廓拟合圆
时间: 2024-09-14 14:01:14 浏览: 41
在Python中使用OpenCV库进行轮廓拟合圆的步骤大致如下:
1. 首先需要安装OpenCV库,可以使用pip安装:
```python
pip install opencv-python
```
2. 导入OpenCV库,并读取需要处理的图像:
```python
import cv2
# 读取图像
image = cv2.imread('path_to_image.jpg')
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
```
3. 对图像进行二值化处理,以便更好地找到轮廓:
```python
_, thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)
```
4. 使用`findContours`函数找到图像中的轮廓:
```python
contours, _ = cv2.findContours(thresh, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
```
5. 遍历找到的轮廓,对每个轮廓使用`minEnclosingCircle`函数拟合最小外接圆:
```python
for cnt in contours:
(x, y), radius = cv2.minEnclosingCircle(cnt)
center = (int(x), int(y))
radius = int(radius)
# 在原图上绘制圆
cv2.circle(image, center, radius, (0, 255, 0), 2)
```
6. 显示图像:
```python
cv2.imshow('Circles', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
以上步骤中,`minEnclosingCircle`函数返回的是圆心坐标和半径。圆心坐标是浮点数,半径也是浮点数,因此在绘制时需要转换为整数类型。
阅读全文
相关推荐
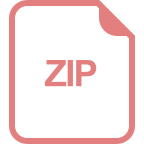
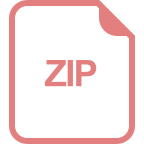
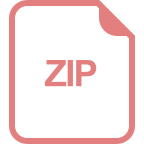






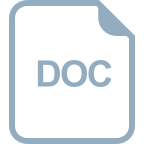
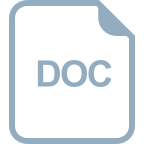
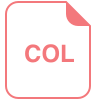






