c语言从键盘输入三行文字(每行不超过80个字符),统计出其中大写字母、小马字母、数字字符、空格、其它字符的个数
时间: 2024-12-12 07:21:54 浏览: 14
在 C 语言中,你可以通过标准库函数如 getchar() 和 isalnum(), isupper(), islower(), isspace() 来实现这个功能。以下是一个简单的示例程序:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
int uppercase = 0;
int lowercase = 0;
int digits = 0;
int spaces = 0;
int others = 0;
char input[3][90]; // 三行最多容纳80个字符加上换行符
for (int i = 0; i < 3; i++) {
fgets(input[i], sizeof(input[i]), stdin); // 输入一行
for (int j = 0; j < strlen(input[i]); j++) {
if (isupper(input[i][j])) { // 判断是否为大写字母
uppercase++;
} else if (islower(input[i][j])) { // 判断是否为小写字母
lowercase++;
} else if (isdigit(input[i][j])) { // 判断是否为数字
digits++;
} else if (isspace(input[i][j])) { // 判断是否为空格
spaces++;
} else { // 其他字符
others++;
}
}
}
printf("大写字母: %d\n", uppercase);
printf("小写字母: %d\n", lowercase);
printf("数字字符: %d\n", digits);
printf("空格: %d\n", spaces);
printf("其他字符: %d\n", others);
return 0;
}
```
在这个程序中,我们首先定义了几个变量分别用于计数各种类型的字符。然后,通过两层循环遍历输入的每一行以及每个字符,判断其类型并累加到相应的计数器上。
阅读全文
相关推荐
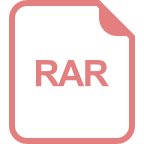
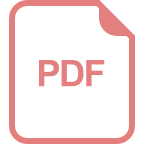
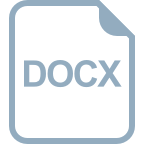
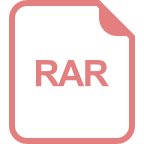
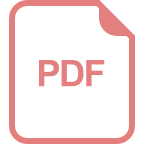
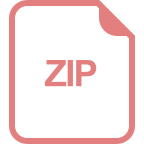
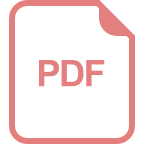
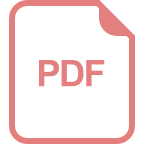
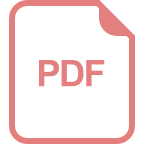
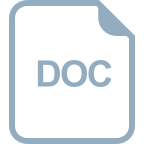
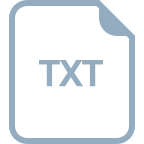