vue2实现防抖节流
时间: 2023-10-19 09:08:08 浏览: 266
在Vue 2中实现防抖和节流的方法是通过自定义指令或者在方法中使用`setTimeout`来实现的。下面分别介绍两种方式的实现:
1. 使用自定义指令实现防抖和节流:
```javascript
// 使用自定义指令 v-debounce
Vue.directive('debounce', {
inserted: function (el, binding) {
let timer = null;
el.addEventListener('input', function () {
if (timer) {
clearTimeout(timer);
}
timer = setTimeout(() => {
binding.value();
}, 500); // 设置延迟时间,单位为毫秒
});
}
});
// 使用自定义指令 v-throttle
Vue.directive('throttle', {
inserted: function (el, binding) {
let timer = null;
el.addEventListener('input', function () {
if (!timer) {
timer = setTimeout(() => {
binding.value();
timer = null;
}, 500); // 设置间隔时间,单位为毫秒
}
});
}
});
```
在模板中使用自定义指令:
```html
<input v-debounce="handleInput" />
<input v-throttle="handleInput" />
```
其中`handleInput`为需要防抖或者节流的方法。
2. 在方法中使用`setTimeout`实现防抖和节流:
```javascript
export default {
data() {
return {
timer: null
}
},
methods: {
debounce(method, delay) {
clearTimeout(this.timer);
this.timer = setTimeout(() => {
method();
}, delay);
},
throttle(method, interval) {
if (!this.timer) {
method();
this.timer = setTimeout(() => {
this.timer = null;
}, interval);
}
},
handleInput() {
// 防抖示例
this.debounce(() => {
// 处理输入事件
}, 500);
// 节流示例
this.throttle(() => {
// 处理输入事件
}, 500);
}
}
}
```
在模板中绑定方法:
```html
<input @input="handleInput" />
```
以上两种方式都可以实现防抖和节流效果,根据具体的需求选择合适的方式即可。
阅读全文
相关推荐
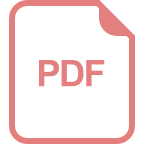










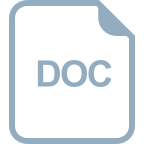



