策略模式代替if else
时间: 2023-08-14 15:14:13 浏览: 55
策略模式是一种行为设计模式,用于将不同的算法或策略封装成独立的类,并使它们可以互相替换,以实现更灵活的代码结构。相比于使用大量的 if-else 语句,策略模式可以提高代码的可读性、可维护性和可扩展性。
在使用策略模式时,您可以创建一个抽象策略类,定义一个共同的接口或抽象方法。然后,针对不同的算法或策略,创建具体的策略类,实现这个共同的接口或抽象方法。最后,在使用时,通过依赖注入或其他方式将具体策略类传递给使用它的对象。
下面是一个示例代码,演示了如何使用策略模式代替 if-else:
```python
# 定义抽象策略类
class Strategy:
def do_operation(self):
pass
# 定义具体策略类
class StrategyA(Strategy):
def do_operation(self):
print("执行策略A")
class StrategyB(Strategy):
def do_operation(self):
print("执行策略B")
class Context:
def __init__(self, strategy):
self.strategy = strategy
def execute_strategy(self):
self.strategy.do_operation()
# 使用
strategy_a = StrategyA()
strategy_b = StrategyB()
context = Context(strategy_a)
context.execute_strategy() # 输出:执行策略A
context.strategy = strategy_b
context.execute_strategy() # 输出:执行策略B
```
在上述示例中,我们定义了一个抽象策略类 `Strategy`,其中包含了一个抽象方法 `do_operation`。然后,我们创建了两个具体策略类 `StrategyA` 和 `StrategyB`,它们分别实现了 `do_operation` 方法。
最后,我们创建了一个 `Context` 类,它接受一个具体策略类作为参数,并在 `execute_strategy` 方法中调用策略类的 `do_operation` 方法来执行具体的策略。
通过这种方式,我们可以根据需要动态地切换和使用不同的策略,而不需要使用大量的 if-else 来处理不同的情况。这提高了代码的可读性和可维护性,并且使得新的策略可以更方便地添加进来。
相关推荐
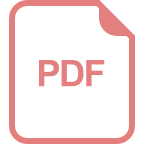
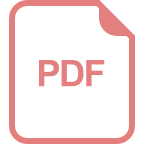














