python遍历文件夹图片
时间: 2023-08-15 12:07:47 浏览: 59
您可以使用Python的os和PIL库来遍历文件夹中的图片。下面是一个示例代码:
```python
import os
from PIL import Image
# 指定要遍历的文件夹路径
folder_path = "your_folder_path"
# 遍历文件夹中的文件
for filename in os.listdir(folder_path):
# 判断文件是否为图片
if filename.endswith(".png") or filename.endswith(".jpg"):
# 图片文件的完整路径
img_path = os.path.join(folder_path, filename)
# 打开图片
img = Image.open(img_path)
# 在这里可以对图片进行处理或进行其他操作
# 例如,输出图片的大小
print("图片名称: {}, 大小: {}x{}".format(filename, img.width, img.height))
```
在上面的示例中,您需要将"your_folder_path"替换为要遍历的文件夹的路径。代码会遍历文件夹中的每个文件,如果文件以".png"或".jpg"结尾,则将其视为图片文件。然后,您可以使用PIL库打开图片并进行处理。以上只是一个简单的示例,您可以根据自己的需求进行进一步操作。
相关问题
python 遍历文件夹图片显示
要Python中遍历文件夹并显示图片,你可以使用以下代码:
```python
import os
import cv2
def show_images_in_folder(folder):
for root, dirs, files in os.walk(folder):
for file in files:
if file.endswith('.jpg') or file.endswith('.png'): # 只处理.jpg和.png格式的图片
file_path = os.path.join(root, file)
img = cv2.imread(file_path)
cv2.imshow('Image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
folder_path = './org'
show_images_in_folder(folder_path)
```
这段代码使用了`os.walk()`函数来遍历文件夹及其子文件夹中的所有文件。然后,通过`cv2.imread()`函数读取图片文件,并使用`cv2.imshow()`函数显示图片。最后,通过`cv2.waitKey(0)`来等待按键输入,`cv2.destroyAllWindows()`来关闭展示窗口。
Python 遍历文件夹
Python遍历文件夹的方法有多种,其中一种常用的方法是使用os模块的listdir函数来获取文件夹下的所有文件和文件夹的名称。然后可以使用isdir函数来判断每个名称对应的路径是否是文件夹,如果是文件夹,则可以进行进一步的递归遍历。以下是一个示例代码:
```python
import os
def scan_folder(folder_path):
files = os.listdir(folder_path)
for file in files:
file_path = os.path.join(folder_path, file)
if os.path.isdir(file_path):
scan_folder(file_path)
else:
print(file_path)
# 调用函数来遍历文件夹
scan_folder("D:\\")
```
这段代码通过递归的方式遍历了指定文件夹下的所有文件,如果遇到文件夹,则会进一步递归遍历该文件夹下的内容,并打印出所有文件的路径。请注意,该代码只会输出文件路径,不会输出文件夹里面的文件夹或文件。如果需要遍历文件夹下的所有文件和文件夹,请参考方法二。
另外,还可以使用os.walk函数来实现文件夹的遍历,该函数会返回一个生成器,可以逐层遍历文件夹下的所有文件和文件夹。以下是一个示例代码:
```python
import os
def scan_folder(folder_path):
for root, dirs, files in os.walk(folder_path):
for file in files:
file_path = os.path.join(root, file)
print(file_path)
# 调用函数来遍历文件夹
scan_folder("D:\\")
```
这段代码使用了os.walk函数来逐层遍历文件夹下的所有文件和文件夹,然后通过循环打印出所有文件的路径。该方法可以输出文件夹里面的文件夹和文件的路径。
总结起来,Python遍历文件夹可以使用os模块的listdir函数结合递归或者使用os.walk函数来实现。这样可以方便地获取文件夹下的所有文件和文件夹,并进行相应的操作。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* [Python---如何使用python遍历文件夹下的所有文件(输出所有文件夹以及文件)](https://blog.csdn.net/m0_59081230/article/details/121320471)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
- *2* *3* [python递归遍历查询文件 & 文件夹](https://blog.csdn.net/weixin_51033461/article/details/123865385)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
相关推荐
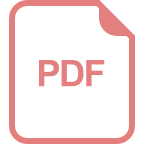
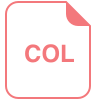
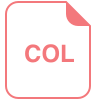








