用python将文件夹当中的手写照片的.jpg文件转换成mnist的文件格式的图片并输入训练完毕的模型里面进行手写字的判断
时间: 2024-09-11 15:04:49 浏览: 63
在Python中,将手写照片的.jpg文件转换为MNIST格式的图片并用于已训练的模型预测,需要经过以下几个步骤:
1. **读取和预处理图片**:
首先,使用PIL库读取.jpg文件,然后进行灰度化、调整大小(如MNIST的28x28像素)以及归一化。这里我们可以参考之前提到的`preprocess_image`函数。
```python
from PIL import Image
import numpy as np
def preprocess_image(image_path):
img = Image.open(image_path)
gray_img = img.convert('L')
resized_img = gray_img.resize((28, 28))
flattened_img = np.array(resized_img).reshape(1, -1) # 添加batch dimension (1, 784)
normalized_img = flattened_img / 255.0
return normalized_img
```
2. **加载训练好的模型**:
如果你已经有一个训练好的MNIST模型(比如TensorFlow的Keras模型或者PyTorch的模型),确保模型能接受1张图片作为输入。例如,对于Keras模型:
```python
import tensorflow as tf
model = load_your_trained_model() # 用load方法加载模型
```
3. **预测和结果处理**:
对于每张预处理后的图片,通过模型进行预测,并根据模型返回的结果判断手写字母。例如,在Keras模型中:
```python
predictions = model.predict(normalized_img)
predicted_label = np.argmax(predictions) # 获取预测的数字标签
```
4. **评估**:
可能还需要一个函数来解码预测的标签回字符,比如:
```python
def decode_prediction(label):
return chr(int(label)) # 假设你的任务是对ASCII字符进行识别
predicted_char = decode_prediction(predicted_label)
print(f"Predicted character: {predicted_char}")
```
5. **批量处理**:
如果有多个.jpg文件,可以使用`os`模块遍历文件夹并逐个处理:
```python
import os
def process_folder(input_folder, output_folder, model):
# ... 其他处理步骤
# ...
for image_filename in os.listdir(input_folder):
if image_filename.endswith(".jpg"):
input_path = os.path.join(input_folder, image_filename)
output_path = os.path.join(output_folder, f"{image_filename.split('.')[0]}_prediction.txt") # 创建输出路径
processed_img = preprocess_image(input_path)
predictions = model.predict(processed_img)
predicted_label = np.argmax(predictions)
predicted_char = decode_prediction(predicted_label)
with open(output_path, "w") as f:
f.write(predicted_char)
```
注意:在实际应用中,你可能需要对模型进行适当的微调,尤其是当你面对的是新领域的手写照片,因为它们可能会有不同的风格或噪声。
阅读全文
相关推荐
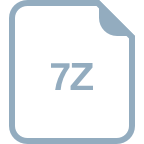
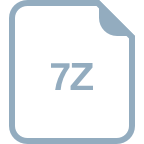
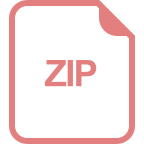
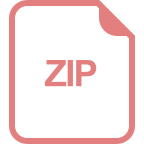
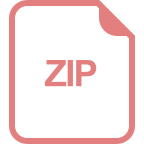
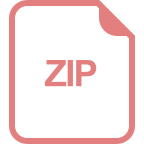
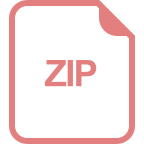
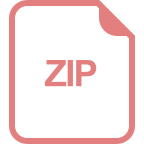
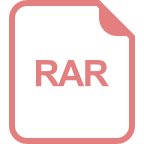
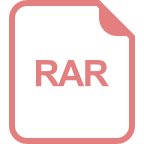
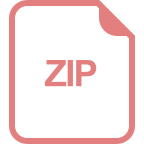
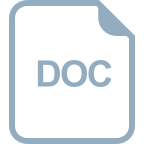






