C自定义print打印函数
时间: 2023-12-22 20:05:27 浏览: 54
C语言中可以通过自定义print打印函数来实现自定义的输出格式和功能。下面是三种实现方式:
1. 利用C库函数printf:
```c
#include <stdio.h>
void myPrint(const char* format, ...) {
va_list args;
va_start(args, format);
vprintf(format, args);
va_end(args);
}
int main() {
myPrint("Hello, %s!\n", "world"); // 输出:Hello, world!
myPrint("The answer is %d.\n", 42); // 输出:The answer is 42.
return 0;
}
```
2. 利用C库函数vsprintf和变参函数:
```c
#include <stdio.h>
#include <stdarg.h>
void myPrint(const char* format, ...) {
char buffer[100];
va_list args;
va_start(args, format);
vsprintf(buffer, format, args);
va_end(args);
printf("%s", buffer);
}
int main() {
myPrint("Hello, %s!\n", "world"); // 输出:Hello, world!
myPrint("The answer is %d.\n", 42); // 输出:The answer is 42.
return 0;
}
```
3. 自行完成参数提取,格式化:
```c
#include <stdio.h>
#include <stdarg.h>
void myPrint(const char* format, ...) {
va_list args;
va_start(args, format);
while (*format != '\0') {
if (*format == '%') {
format++;
if (*format == 's') {
char* str = va_arg(args, char*);
printf("%s", str);
} else if (*format == 'd') {
int num = va_arg(args, int);
printf("%d", num);
}
} else {
printf("%c", *format);
}
format++;
}
va_end(args);
}
int main() {
myPrint("Hello, %s!\n", "world"); // 输出:Hello, world!
myPrint("The answer is %d.\n", 42); // 输出:The answer is 42.
return 0;
}
```
相关推荐













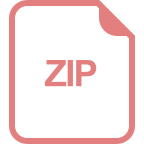
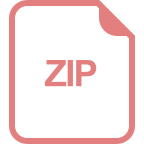