qt实现udp网络定时通信
时间: 2024-11-06 22:14:37 浏览: 58
在Qt中实现UDP网络的定时通信,你可以使用QDatagramSocket类和QTimer类来完成。以下是简单的步骤:
1. **设置依赖**:
首先,在你的Qt项目中,需要包含`<QtNetwork/QTcpSocket>`和`<QtCore/QTimer>`头文件。
2. **创建socket**:
创建一个`QUdpSocket`实例,它是用于UDP通信的,然后初始化它并绑定到指定的端口。
```cpp
QUdpSocket* udpSocket = new QUdpSocket(this);
if (!udpSocket->bind(QHostAddress::Any, yourPort)) {
qDebug() << "Failed to bind UDP socket";
}
```
3. **设置定时发送**:
使用`QTimer`定时发送数据包。例如,每间隔一定时间,发送一个预先准备好的UDP数据报文。
```cpp
QTimer* timer = new QTimer(this);
connect(timer, &QTimer::timeout, [this, udpSocket](){
QByteArray sendData;
//...填充你要发送的数据
udpSocket->write(sendData);
});
timer->start(intervalInMilliseconds); // 设置发送间隔
```
4. **接收数据**:
如果你也想接收数据,可以使用`readyRead()`信号连接到槽函数,处理接收到的数据。
```cpp
connect(udpSocket, &QUdpSocket::readyRead, this, &YourClass::handleReceivedDatagrams);
```
5. **处理异常**:
当然,别忘了处理可能出现的错误,如套接字失败、超时等,并在适当的时候关闭socket。
```cpp
void YourClass::closeSocket()
{
if (udpSocket)
{
udpSocket->deleteLater();
udpSocket = nullptr;
}
}
```
阅读全文
相关推荐






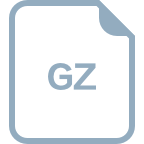

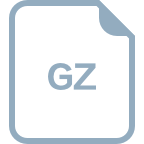








