C#post请求中 传入数组类型的body 使用Newtonsoft.Json
时间: 2023-12-06 13:27:46 浏览: 53
可以使用下面的代码来实现C#中的POST请求传递数组类型的body,使用Newtonsoft.Json进行序列化和反序列化:
```csharp
using Newtonsoft.Json;
using System;
using System.Collections.Generic;
using System.IO;
using System.Net;
using System.Text;
public class Program
{
static void Main(string[] args)
{
// 定义POST请求的URL地址
string url = "http://example.com/api/test";
// 定义POST请求的Body参数,这里使用List<string>类型的数组
List<string> data = new List<string>()
{
"value1",
"value2",
"value3"
};
// 将List<string>类型的数组转换为JSON格式的字符串
string jsonData = JsonConvert.SerializeObject(data);
// 设置POST请求的Header参数
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(url);
request.Method = "POST";
request.ContentType = "application/json";
// 将JSON格式的字符串转换为Byte数组
byte[] byteData = Encoding.UTF8.GetBytes(jsonData);
// 设置POST请求的Body参数
request.ContentLength = byteData.Length;
Stream requestStream = request.GetRequestStream();
requestStream.Write(byteData, 0, byteData.Length);
requestStream.Close();
// 发送POST请求并接收响应
HttpWebResponse response = (HttpWebResponse)request.GetResponse();
Stream responseStream = response.GetResponseStream();
StreamReader reader = new StreamReader(responseStream);
string responseText = reader.ReadToEnd();
Console.WriteLine(responseText);
reader.Close();
responseStream.Close();
response.Close();
}
}
```
在上面的代码中,我们定义了一个List<string>类型的数组,然后使用Newtonsoft.Json将其转换为JSON格式的字符串。接着,我们设置了POST请求的Header参数,包括请求的方法、请求的Content-Type和请求的Body参数。最后,我们发送POST请求并接收响应,将响应输出到控制台上。
相关推荐
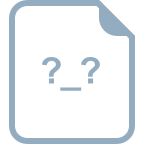














