matlab app实现串口发送和接收完整代码
时间: 2023-06-26 10:09:51 浏览: 71
以下是一个基本的 MATLAB App 示例,演示如何使用串口进行数据发送和接收。在这个例子中,我们将从串口接收数据,并将其显示在 GUI 中的文本框中,同时我们也可以将文本框中输入的数据发送到串口。
```matlab
classdef SerialComm < matlab.apps.AppBase
% Properties that correspond to app components
properties (Access = public)
UIFigure matlab.ui.Figure
TextArea matlab.ui.control.TextArea
SendButton matlab.ui.control.Button
TextField matlab.ui.control.EditField
end
% Properties that correspond to app components
properties (Access = private)
SerialPort % 串口对象
end
% Callbacks that handle component events
methods (Access = private)
% Button pushed function: SendButton
function SendButtonPushed(app, event)
% 从文本框中获取数据
data = app.TextField.Value;
% 将数据写入串口
fprintf(app.SerialPort, data);
end
% Serial port data available event
function SerialPortDataAvailable(app, event)
% 读取串口数据
data = readline(app.SerialPort);
% 将数据显示在文本框中
app.TextArea.Value = data;
end
end
% App initialization and construction
methods (Access = private)
% Create UIFigure and components
function createComponents(app)
% Create UIFigure
app.UIFigure = uifigure;
app.UIFigure.Position = [100 100 640 480];
app.UIFigure.Name = 'SerialComm';
app.UIFigure.Resize = 'off';
% Create TextArea
app.TextArea = uitextarea(app.UIFigure);
app.TextArea.Position = [20 60 600 380];
% Create SendButton
app.SendButton = uibutton(app.UIFigure, 'push');
app.SendButton.ButtonPushedFcn = createCallbackFcn(app, @SendButtonPushed, true);
app.SendButton.Position = [400 20 100 22];
app.SendButton.Text = 'Send';
% Create TextField
app.TextField = uieditfield(app.UIFigure, 'text');
app.TextField.Position = [20 20 360 22];
app.TextField.Value = 'Enter Text Here';
end
% Create serial port object
function createSerialPort(app)
% Configure and open serial port
app.SerialPort = serialport('COM1', 9600);
configureTerminator(app.SerialPort, "LF");
app.SerialPort.UserData = struct("DataAvailableFcn",...
@SerialPortDataAvailable);
% Register serial port data available event
configureCallback(app.SerialPort, "DataAvailable", ...
app.SerialPort.UserData.DataAvailableFcn);
% Open serial port
fopen(app.SerialPort);
end
end
% App startup and shutdown
methods (Access = protected)
% App startup function: startupFcn
function startupFcn(app)
% 创建UI组件
createComponents(app);
% 创建串口对象
createSerialPort(app);
end
% App shutdown function: shutdownFcn
function shutdownFcn(app)
% 关闭串口
fclose(app.SerialPort);
% 删除串口对象
delete(app.SerialPort);
end
end
% Component initialization
methods (Access = private)
% Initialize app components
function initComponents(app)
end
end
% Create app
methods (Access = public)
% Construct app
function app = SerialComm
% Create and configure components
createComponents(app);
% Register the app with App Designer
registerApp(app, app.UIFigure);
% Execute the startup function
runStartupFcn(app, @startupFcn);
if nargout == 0
clear app
end
end
end
end
```
在这个示例中,我们创建了一个 App,其中包含了一个 TextArea 和一个 EditField,以及一个 SendButton。我们还创建了一个串口对象SerialPort,用于与串口进行通信。
在我们的 App 中,当用户点击发送按钮时,我们从 EditField 中获取数据,然后将其写入串口。当串口接收到数据时,我们从串口读取数据,并将其显示在 TextArea 中。
在我们的 App 的 startupFcn 函数中,我们创建了 UI 组件,并创建了串口对象。在 shutdownFcn 函数中,我们关闭了串口并删除了串口对象。
在上面的示例中,我们使用 COM1 端口和 9600 波特率。您需要根据您的实际情况更改这些值。
相关推荐
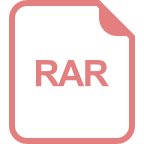














