网页上传商品的后端代码java怎么写
时间: 2023-12-03 07:45:23 浏览: 73
上传的后端代码需要实现以下步骤:
1. 接收上传的商品信息,包括商品名称、描述、价格、图片等。
2. 将商品信息保存到数据库中,可以使用ORM框架如MyBatis或Hibernate。
3. 上传图片到服务器,可以使用第三方库如Apache Commons FileUpload或Spring的MultipartResolver来处理文件上传。
以下是一个简单的Java代码示例,展示了如何上传商品信息和图片:
```java
@PostMapping("/product")
public ResponseEntity<String> uploadProduct(@RequestParam("name") String name,
@RequestParam("description") String description,
@RequestParam("price") double price,
@RequestParam("image") MultipartFile image) {
// 保存商品信息到数据库
Product product = new Product(name, description, price);
productService.saveProduct(product);
// 上传图片到服务器
String filename = StringUtils.cleanPath(image.getOriginalFilename());
try {
if (image.isEmpty()) {
throw new RuntimeException("Failed to upload empty file.");
}
if (filename.contains("..")) {
throw new RuntimeException("Cannot upload file with relative path outside current directory.");
}
Path targetPath = Paths.get(uploadPath + filename);
Files.copy(image.getInputStream(), targetPath, StandardCopyOption.REPLACE_EXISTING);
} catch (IOException e) {
throw new RuntimeException("Failed to upload file.", e);
}
return ResponseEntity.ok("Product uploaded successfully.");
}
```
在此示例中,我们使用Spring Boot框架来实现后端接口,并通过`@RequestParam`注解来接收上传的商品信息和图片。其中,`MultipartFile`对象表示上传的文件,可以通过其`getInputStream`方法来获取文件内容。
在保存商品信息到数据库时,我们可以使用ORM框架如MyBatis或Hibernate来简化数据库操作。此处我们假设已经定义了一个名为`ProductService`的服务,其中的`saveProduct`方法用于保存商品信息到数据库。
上传文件到服务器时,我们需要使用`Files.copy`方法将文件内容写入服务器文件系统中。在此示例中,我们将上传的文件保存到了一个名为`uploadPath`的目录中。为了避免文件名中包含非法字符,我们使用了`StringUtils.cleanPath`方法来清理文件名。
需要注意的是,以上示例仅为演示用途,实际应用中可能需要进行更多的错误检查和安全措施。
阅读全文
相关推荐
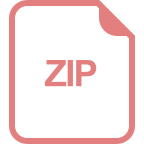



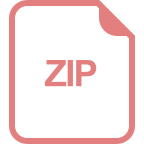
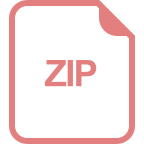
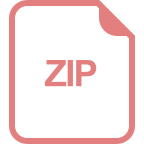
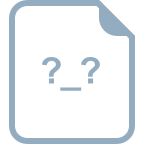
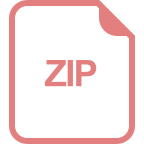
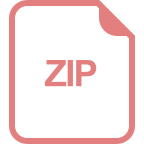
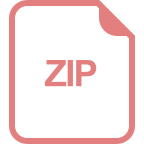
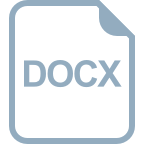
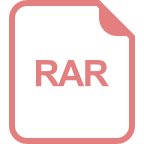
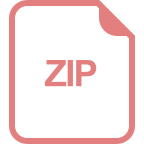
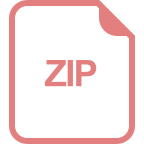
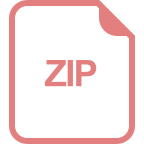