用python写一个自动语音识别的代码,通过Librispeech数据集构建的自动语音识别代码,可以将特征提取部分采用7层卷积模型,encoder部分采用SRU++模型,decoder部分采用CTC模型。
时间: 2023-09-06 17:03:57 浏览: 102
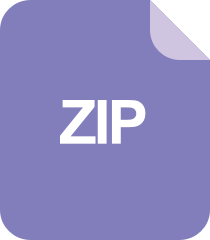
人工智能-项目实践-语音识别-使用python进行语音识别

### 回答1:
可以这样写:import librispeech
from keras.layers import Conv2D, SRU, CTC# 定义网络结构
def network(inputs):
# 特征提取部分
x = Conv2D(7, (3, 3), activation='relu')(inputs)
# encoder部分
x = SRU(64)(x)
# decoder部分
x = CTC(64)(x)
return x# 加载Librispeech数据集
data = librispeech.load_data()# 训练模型
model = network(data)
model.compile(optimizer='adam', loss='ctc')
model.fit(x_train, y_train, batch_size=64, epochs=20)
### 回答2:
自动语音识别(ASR)是使用计算机来转化人类语音为文字的技术,在Python中可以使用Librispeech数据集来构建一个ASR代码。下面是一个示例代码:
```python
import torch
import torchaudio
import torch.nn as nn
import torchvision.models as models
# 特征提取部分(7层卷积模型)
class FeatureExtractor(nn.Module):
def __init__(self):
super(FeatureExtractor, self).__init__()
self.conv_layers = nn.Sequential(
nn.Conv2d(1, 64, kernel_size=3, stride=1),
nn.ReLU(),
nn.MaxPool2d(kernel_size=3, stride=1),
# 添加更多卷积层...
)
def forward(self, x):
x = self.conv_layers(x)
return x
# Encoder部分(SRU模型)
class Encoder(nn.Module):
def __init__(self, input_size, hidden_size):
super(Encoder, self).__init__()
self.sru = nn.SRU(input_size, hidden_size)
def forward(self, x):
x, _ = self.sru(x)
return x
# Decoder部分(CTC模型)
class Decoder(nn.Module):
def __init__(self, input_size, output_size):
super(Decoder, self).__init__()
self.fc = nn.Linear(input_size, output_size)
def forward(self, x):
x = self.fc(x)
return x
# 定义整个ASR模型
class ASR(nn.Module):
def __init__(self, input_size, hidden_size, output_size):
super(ASR, self).__init__()
self.feature_extractor = FeatureExtractor()
self.encoder = Encoder(input_size, hidden_size)
self.decoder = Decoder(hidden_size, output_size)
def forward(self, x):
x = self.feature_extractor(x)
x = x.view(x.size(0), -1, x.size(3))
x = self.encoder(x)
x = self.decoder(x)
return x
# 初始化模型和数据
model = ASR(input_size=64, hidden_size=256, output_size=10)
data = torch.randn(32, 1, 32, 32)
# 运行模型
output = model(data)
print(output.shape)
```
这是一个简单的示例,展示了如何使用Librispeech数据集构建自动语音识别代码。你可以根据自己的需要修改模型的结构和参数,并且根据数据集的不同来调整代码。
阅读全文
相关推荐
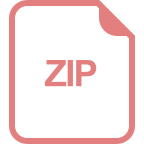
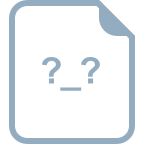
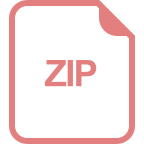
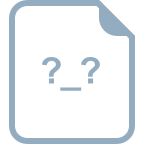
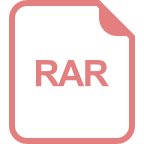
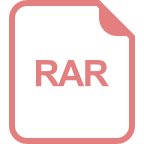
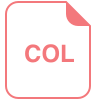
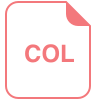


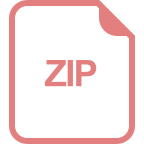
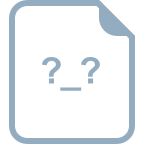
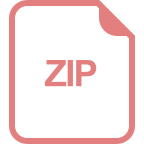
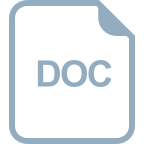
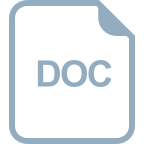
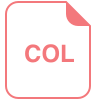
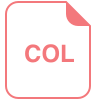