写一个菜单程序,能够对动物园的动物进行管理 动物类 (id. type、 color、sex、price、buyDate(入园时间)、 isDead=false) 」、添加动物信息进动物园 2、注销一条动物信息(死亡或卖掉) 3、查询全部动物信息 4、查询某种颜色的动物信息 5、統计某种类型的动物信息 6、统计某种类型的动物的价值 7、修改某个动物的基本信息(输入id) 8、退出 注!:注销动物信息,可以通过改变动物的某一个特殊属性来控制。 比如,动物有个属性叫 isDead,正常情况下是 false,当注销的时候改成 true,然后其他所有的统计和查询都只列出 isDead 属性为 false 的动物来,这样,看起来这个动物就不存在了。 注2:id 是用来唯一标识动物信息的,区别动物主要就得靠ID了,所以添加动物信息的时候,id 不允许重复 注3:动物信息是指类似下面的信息: ID:3、老虎、白色、雌、370000购买、2012-3-28入园
时间: 2023-12-31 08:03:38 浏览: 95
好的,这是一个比较完整的菜单程序,可以对动物园的动物进行管理。代码如下(注释已加):
```python
# 定义动物类
class Animal:
def __init__(self, id, type, color, sex, price, buyDate):
self.id = id
self.type = type
self.color = color
self.sex = sex
self.price = price
self.buyDate = buyDate
self.isDead = False
# 定义动物园类
class Zoo:
def __init__(self):
self.animals = []
# 添加动物信息
def addAnimal(self, id, type, color, sex, price, buyDate):
# 判断id是否已存在
for animal in self.animals:
if animal.id == id:
print("该动物已存在!")
return
# 创建新动物实例
newAnimal = Animal(id, type, color, sex, price, buyDate)
# 添加至动物园
self.animals.append(newAnimal)
print("添加成功!")
# 注销一条动物信息(死亡或卖掉)
def cancelAnimal(self, id):
# 判断id是否存在
for animal in self.animals:
if animal.id == id:
# 修改isDead属性
animal.isDead = True
print("注销成功!")
return
print("该动物不存在!")
# 查询全部动物信息
def queryAll(self):
print("所有动物信息:")
for animal in self.animals:
# 判断isDead属性
if not animal.isDead:
print("ID:{}, {}, {}, {}, {}购买, {}入园".format(animal.id, animal.type, animal.color, animal.sex, animal.price, animal.buyDate))
# 查询某种颜色的动物信息
def queryByColor(self, color):
print("颜色为{}的动物信息:".format(color))
for animal in self.animals:
# 判断isDead属性和颜色
if not animal.isDead and animal.color == color:
print("ID:{}, {}, {}, {}, {}购买, {}入园".format(animal.id, animal.type, animal.color, animal.sex, animal.price, animal.buyDate))
# 統計某种类型的动物信息
def countByType(self, type):
count = 0
for animal in self.animals:
# 判断isDead属性和类型
if not animal.isDead and animal.type == type:
count += 1
print("类型为{}的动物数量为{}".format(type, count))
# 统计某种类型的动物的价值
def countPriceByType(self, type):
price = 0
for animal in self.animals:
# 判断isDead属性和类型
if not animal.isDead and animal.type == type:
price += animal.price
print("类型为{}的动物总价值为{}".format(type, price))
# 修改某个动物的基本信息(输入id)
def modifyAnimal(self, id):
# 判断id是否存在
for animal in self.animals:
if animal.id == id:
# 修改属性
animal.color = input("请输入新颜色:")
animal.price = int(input("请输入新价格:"))
print("修改成功!")
return
print("该动物不存在!")
# 菜单程序
def menu(self):
while True:
print("动物园管理系统")
print("1.添加动物信息")
print("2.注销动物信息")
print("3.查询全部动物信息")
print("4.查询某种颜色的动物信息")
print("5.统计某种类型的动物信息")
print("6.统计某种类型的动物的价值")
print("7.修改某个动物的基本信息")
print("8.退出")
choice = input("请输入要进行的操作:")
if choice == "1":
id = int(input("请输入动物ID:"))
type = input("请输入动物类型:")
color = input("请输入动物颜色:")
sex = input("请输入动物性别:")
price = int(input("请输入动物价格:"))
buyDate = input("请输入动物入园时间:")
self.addAnimal(id, type, color, sex, price, buyDate)
elif choice == "2":
id = int(input("请输入动物ID:"))
self.cancelAnimal(id)
elif choice == "3":
self.queryAll()
elif choice == "4":
color = input("请输入颜色:")
self.queryByColor(color)
elif choice == "5":
type = input("请输入类型:")
self.countByType(type)
elif choice == "6":
type = input("请输入类型:")
self.countPriceByType(type)
elif choice == "7":
id = int(input("请输入动物ID:"))
self.modifyAnimal(id)
elif choice == "8":
print("谢谢使用,再见!")
break
else:
print("输入有误,请重新输入!")
# 测试
zoo = Zoo()
zoo.menu()
```
使用时,可通过调用菜单程序来实现对动物园动物信息的管理。例如,输入1添加动物信息,输入3查询全部动物信息。
阅读全文
相关推荐
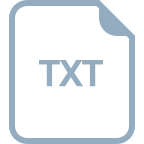

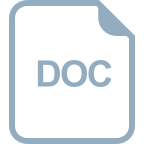
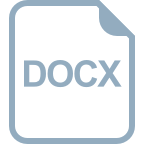
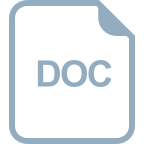
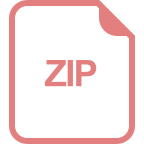
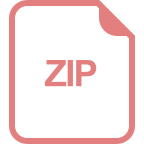
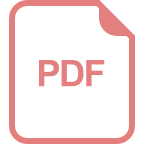
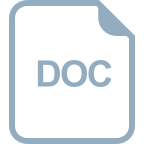
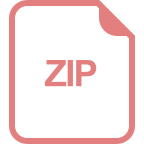
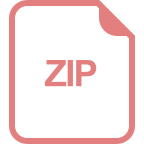
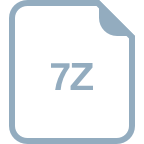
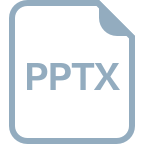
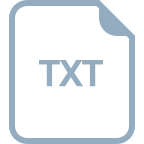
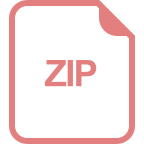