C语言实现一段数据存入bin文件中
时间: 2024-10-13 22:13:27 浏览: 37
在C语言中,你可以使用标准库函数`fwrite()`来将数据存储到二进制文件(`.bin`)中。下面是一个简单的例子,假设我们有一个结构体`Person`,并且想要将它的实例写入文件:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义一个简单的结构体
struct Person {
char name[50];
int age;
};
void write_to_bin_file(const char* filename, struct Person person) {
// 打开文件(如果不存在则创建),以二进制模式("wb")打开
FILE* file = fopen(filename, "wb");
if (file == NULL) {
perror("Failed to open file");
return;
}
// 将结构体成员转换为字节并写入文件
fwrite(person.name, sizeof(char), 50, file); // 名字部分
fwrite(&person.age, sizeof(int), 1, file); // 年龄部分
// 关闭文件
fclose(file);
}
// 使用示例
int main() {
struct Person p = {"Alice", 30};
const char* filepath = "data.bin";
write_to_bin_file(filepath, p);
printf("Data written successfully.\n");
return 0;
}
```
当你运行这个程序,它会把`Person`结构体的信息(名字和年龄)保存到名为"data.bin"的文件中。
阅读全文
相关推荐
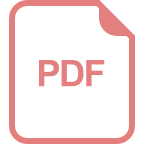
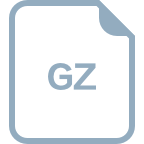
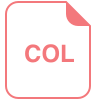
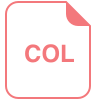
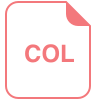
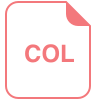
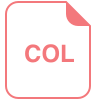
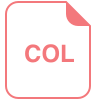
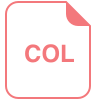

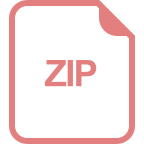