用python写一个A*算法解决八代码问题
时间: 2024-05-09 17:16:42 浏览: 12
好的,下面是用Python实现A*算法解决八数码问题的示例代码:
```python
import heapq
class State:
def __init__(self, board, moves=0, goal=None):
self.board = board
self.moves = moves
self.goal = goal
def __lt__(self, other):
return (self.moves + self.heuristic()) < (other.moves + other.heuristic())
def heuristic(self):
if self.goal is None:
return 0
return sum(abs(self.board[i] // 3 - self.goal[i] // 3) + abs(self.board[i] % 3 - self.goal[i] % 3) for i in range(9))
def __eq__(self, other):
return self.board == other.board
def __hash__(self):
return hash(str(self.board))
class Solver:
def __init__(self, start, goal):
self.start = State(start, goal=goal)
self.goal = State(goal)
self.visited = set()
def solve(self):
queue = []
heapq.heappush(queue, self.start)
while queue:
state = heapq.heappop(queue)
if state == self.goal:
return state.moves
self.visited.add(state)
for neighbor in self.neighbors(state):
if neighbor not in self.visited:
heapq.heappush(queue, neighbor)
return -1
def neighbors(self, state):
neighbors = []
i = state.board.index(0)
if i in [3, 4, 5, 6, 7, 8]:
copy = state.board[:]
copy[i], copy[i - 3] = copy[i - 3], copy[i]
neighbors.append(State(copy, state.moves + 1, self.goal.board))
if i in [0, 1, 2, 3, 4, 5]:
copy = state.board[:]
copy[i], copy[i + 3] = copy[i + 3], copy[i]
neighbors.append(State(copy, state.moves + 1, self.goal.board))
if i in [1, 2, 4, 5, 7, 8]:
copy = state.board[:]
copy[i], copy[i - 1] = copy[i - 1], copy[i]
neighbors.append(State(copy, state.moves + 1, self.goal.board))
if i in [0, 1, 3, 4, 6, 7]:
copy = state.board[:]
copy[i], copy[i + 1] = copy[i + 1], copy[i]
neighbors.append(State(copy, state.moves + 1, self.goal.board))
return neighbors
start = [1, 2, 3, 0, 4, 6, 7, 5, 8] # 初始状态
goal = [0, 1, 2, 3, 4, 5, 6, 7, 8] # 目标状态
solver = Solver(start, goal)
print(solver.solve()) # 输出解决八数码问题所需的最小步数
```
在这个示例代码中,`State` 类表示一个状态,包括棋盘布局、移动次数和目标状态。`Solver` 类是八数码问题的求解器,它使用 A* 算法来搜索最优解。在 `Solver` 类中,`solve` 方法实现了 A* 算法的主要逻辑,`neighbors` 方法返回当前状态的所有邻居状态。在 `State` 类中,`heuristic` 方法计算当前状态到目标状态的估价函数值。`__lt__` 方法和 `__hash__` 方法用于比较和哈希状态。
在示例代码中,我们使用初始状态和目标状态来创建 `Solver` 类的实例,并调用 `solve` 方法来求解八数码问题。最后,程序输出解决八数码问题所需的最小步数。
相关推荐
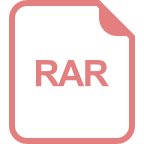
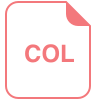
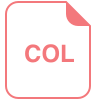
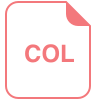
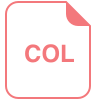
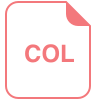









