写一段Python爬虫代码要求使用bs4
时间: 2023-09-05 13:13:53 浏览: 97
好的,以下是一段使用bs4的Python爬虫代码,可以爬取指定网站的所有链接:
```python
import requests
from bs4 import BeautifulSoup
# 定义要爬取的网站链接
url = 'https://www.example.com'
# 发送get请求获取页面内容
response = requests.get(url)
# 使用BeautifulSoup解析页面内容
soup = BeautifulSoup(response.text, 'html.parser')
# 获取所有链接标签<a>,并打印出链接地址
for link in soup.find_all('a'):
print(link.get('href'))
```
希望能对您有所帮助!
相关问题
写一段python爬虫代码
好的,这是一段简单的 Python 爬虫代码,它使用了 requests 库来发送 HTTP 请求并获取网页的内容,使用 BeautifulSoup 库来解析 HTML 标签:
```python
import requests
from bs4 import BeautifulSoup
# 设置要爬取的网页地址
url = 'http://www.example.com'
# 发送 HTTP 请求并获取响应
response = requests.get(url)
# 解析网页内容
soup = BeautifulSoup(response.text, 'html.parser')
# 获取所有的 <a> 标签
links = soup.find_all('a')
# 遍历 <a> 标签并打印链接文本和地址
for link in links:
print(link.text, link['href'])
```
这段代码会发送一个 HTTP 请求到给定的 URL,然后解析响应中的 HTML 标签,最后遍历所有的 <a> 标签并打印出链接文本和地址。
希望这能帮到你!
写一段 Python 爬虫代码
### 回答1:
我可以回答这个问题。以下是一个简单的 Python 爬虫代码示例:
```python
import requests
from bs4 import BeautifulSoup
url = 'https://www.example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# 打印页面标题
print(soup.title.text)
```
这个代码使用 requests 库发送 HTTP 请求,然后使用 BeautifulSoup 库解析 HTML 页面。最后,它打印出页面的标题。当然,这只是一个简单的示例,实际的爬虫代码可能会更复杂。
### 回答2:
下面是一个简单的Python爬虫代码示例:
```python
import requests
from bs4 import BeautifulSoup
def spider(url):
# 发送HTTP GET请求获取页面内容
response = requests.get(url)
# 使用BeautifulSoup解析页面内容
soup = BeautifulSoup(response.text, 'html.parser')
# 在页面中定位要爬取的数据
data = soup.find('div', {'class': 'data-container'}).text
# 输出爬取到的数据
print(data)
# 调用爬虫函数并传入要爬取的页面URL
spider('https://example.com')
```
以上代码使用Python的第三方库requests发送一个HTTP GET请求来获取页面内容,并使用BeautifulSoup解析页面内容。然后通过定位页面中的某个元素,提取到要爬取的数据,并将其打印输出。
在这个示例中,我们使用了一个模拟的URL(https://example.com),你可以根据你想爬取的具体页面来修改URL,并根据页面结构和要提取的数据更改代码中的定位方式。
请注意,爬取网页内容时需要遵守相关法律法规和网站的规定,确保你的爬虫代码不会对网站造成不良影响。
### 回答3:
以下是一个简单的Python爬虫代码示例:
```python
import requests
from bs4 import BeautifulSoup
# 爬取网页内容
url = "https://example.com"
response = requests.get(url)
soup = BeautifulSoup(response.text, "html.parser")
# 提取网页元素
title = soup.find("h1").text
paragraphs = soup.find_all("p")
# 输出结果
print("网页标题: ", title)
print("网页段落:")
for p in paragraphs:
print(p.text)
```
这段代码使用了requests库来发送HTTP请求获取网页内容,并使用BeautifulSoup库来解析网页。我们首先指定目标网页的URL,并使用get方法发送get请求。然后,我们使用BeautifulSoup将获取的网页内容转换为BeautifulSoup对象,以便于后续处理。
在这个示例中,我们假设目标网页中有一个标题元素(h1标签)和多个段落元素(p标签)。我们使用find方法获取第一个h1元素,并使用.text属性获取元素的文本内容。然后,我们使用find_all方法获取所有的p元素,并使用.text属性逐个获取每个元素的文本内容。
最后,我们使用print语句输出结果。在这个示例中,我们输出了网页的标题和段落内容。实际使用时,你可以根据需要对代码进行修改,以适应不同的爬取需求。
阅读全文
相关推荐
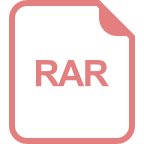
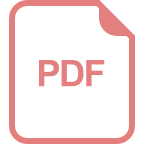








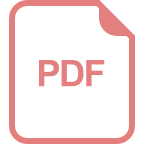
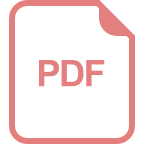
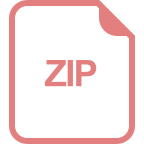
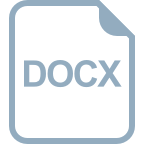