hutool发送http请求
时间: 2023-09-01 17:06:41 浏览: 186
Hutool是一个Java工具库,可以使用它来发送HTTP请求。其中,Hutool的HttpUtil类提供了发送HTTP请求的方法。
以下是使用Hutool发送HTTP请求的示例代码:
```java
import cn.hutool.http.HttpUtil;
public class HttpTest {
public static void main(String[] args) {
// 发送 GET 请求
String result = HttpUtil.get("https://www.baidu.com");
System.out.println(result);
// 发送 POST 请求
String postData = "name=John&age=20";
result = HttpUtil.post("https://example.com/api", postData);
System.out.println(result);
}
}
```
在上面的示例代码中,我们分别使用HttpUtil的get和post方法发送了GET和POST请求,并输出了响应结果。需要注意的是,发送POST请求时,需要将请求参数放在postData字符串中。
相关问题
hutool 发送http 请求
好的,我来给你介绍如何使用 hutool 库发送 HTTP 请求。
首先,你需要在你的项目中添加 hutool 的依赖,你可以在 Maven 项目中添加如下依赖:
```
<dependency>
<groupId>cn.hutool</groupId>
<artifactId>hutool-all</artifactId>
<version>5.2.6</version>
</dependency>
```
然后,你就可以使用 hutool 的 `HttpUtil` 工具类发送 HTTP 请求了。
下面是一个简单的例子,展示了如何使用 `HttpUtil.get` 方法发送一个 GET 请求:
```java
String url = "https://www.example.com";
String result = HttpUtil.get(url);
```
你也可以使用 `HttpUtil.post` 方法发送 POST 请求,这个方法接受两个参数:URL 和请求体内容(可以是字符串、字节数组或者文件)。
例如,你可以使用如下代码发送一个带有请求体的 POST 请求:
```java
String url = "https://www.example.com/post";
String data = "hello world";
String result = HttpUtil.post(url, data);
```
hutool 还提供了很多其他方法,例如可以设置请求头、代理、超时时间等等,你可以参考官方文档了解更多内容。
hutool 发送http请求
你可以使用Hutool库来发送HTTP请求。Hutool是一个Java工具包,提供了丰富的工具方法和简化开发的功能。
下面是使用Hutool发送HTTP请求的示例代码:
```java
import cn.hutool.http.HttpRequest;
import cn.hutool.http.HttpResponse;
import cn.hutool.http.HttpStatus;
public class HttpExample {
public static void main(String[] args) {
// 发送GET请求
HttpResponse response = HttpRequest.get("http://www.example.com").execute();
if (response.getStatus() == HttpStatus.HTTP_OK) {
String result = response.body();
System.out.println(result);
}
// 发送POST请求
String postData = "name=John&age=25";
HttpResponse postResponse = HttpRequest.post("http://www.example.com")
.body(postData)
.execute();
if (postResponse.getStatus() == HttpStatus.HTTP_OK) {
String result = postResponse.body();
System.out.println(result);
}
}
}
```
上面的示例代码中,首先使用`HttpRequest.get()`方法发送了一个GET请求,并使用`execute()`方法执行请求,返回一个`HttpResponse`对象。然后通过判断返回的状态码是否为HTTP_OK(200)来判断请求是否成功,如果成功,则可以通过`body()`方法获取响应体的内容。
发送POST请求与发送GET请求类似,只需使用`HttpRequest.post()`方法,并使用`body()`方法设置POST请求的参数。
需要注意的是,在使用Hutool发送HTTP请求前,需要在项目中引入Hutool依赖。
阅读全文
相关推荐
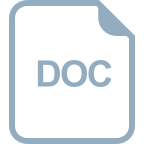
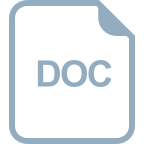
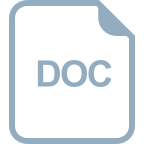













