.net core webapi 上传文件
时间: 2023-04-28 07:05:57 浏览: 176
.NET Core WebAPI 可以通过以下步骤实现文件上传:
1. 在 WebAPI 控制器中添加一个方法,该方法接收一个 IFormFile 类型的参数,用于接收上传的文件。
2. 在方法中使用 IFormFile.SaveAsAsync() 方法将文件保存到指定的位置。
3. 在 WebAPI 的 Startup.cs 文件中添加以下代码,以启用文件上传功能:
```csharp
services.AddMvc(options =>
{
options.Filters.Add(new ConsumesAttribute("multipart/form-data"));
options.Filters.Add(new ProducesAttribute("application/json"));
}).AddJsonOptions(options =>
{
options.SerializerSettings.ContractResolver = new CamelCasePropertyNamesContractResolver();
});
services.Configure<IISServerOptions>(options =>
{
options.AllowSynchronousIO = true;
});
services.Configure<FormOptions>(options =>
{
options.MemoryBufferThreshold = int.MaxValue;
options.ValueLengthLimit = int.MaxValue;
options.MultipartBodyLengthLimit = int.MaxValue;
});
```
4. 在 WebAPI 的控制器方法中使用 [FromForm] 属性将上传的文件绑定到方法参数中。
例如:
```csharp
[HttpPost]
public async Task<IActionResult> UploadFile([FromForm] IFormFile file)
{
if (file == null || file.Length == 0)
{
return BadRequest("File is empty.");
}
var filePath = Path.Combine(_environment.ContentRootPath, "uploads", file.FileName);
using (var stream = new FileStream(filePath, FileMode.Create))
{
await file.CopyToAsync(stream);
}
return Ok("File uploaded successfully.");
}
```
以上就是 .NET Core WebAPI 上传文件的基本步骤。
阅读全文
相关推荐
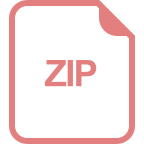
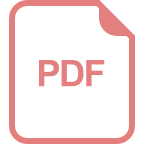















