如何 使用 js 高效的查找出 两个日期间的所有月份,如start=20210331,end=20230615, 找出其中间如 202104,202105... 202306 的所有月份,用数组返回
时间: 2024-03-17 15:45:28 浏览: 12
可以使用 JavaScript 中的 `Date` 对象和循环语句来实现查找两个日期间的所有月份。具体实现方法如下:
1. 将开始日期和结束日期转换为 `Date` 对象。
2. 定义一个数组 `months` 来存储所有的月份。
3. 使用循环语句依次遍历开始日期和结束日期之间的每个月份,并将其添加到 `months` 数组中。
4. 返回 `months` 数组。
以下是示例代码:
```javascript
function getMonthsBetweenDates(start, end) {
const startDate = new Date(start);
const endDate = new Date(end);
const months = [];
let currentDate = startDate;
while (currentDate <= endDate) {
const year = currentDate.getFullYear();
const month = currentDate.getMonth() + 1; // 月份从 0 开始,需要加 1
months.push(`${year}${month.toString().padStart(2, '0')}`);
// 将日期加 1 个月
currentDate.setMonth(currentDate.getMonth() + 1);
}
return months;
}
const start = '20210331';
const end = '20230615';
const months = getMonthsBetweenDates(start, end);
console.log(months);
```
上述代码中,我们使用 `getFullYear()` 和 `getMonth()` 方法获取年份和月份,并使用字符串模板和 `padStart()` 方法将月份转换为两位数格式。然后,我们使用 `setMonth()` 方法将日期加 1 个月,直到遍历完所有的月份。最后,我们返回存储所有月份的 `months` 数组。
相关推荐
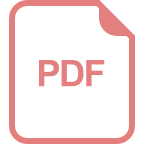
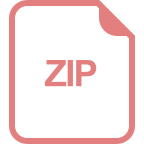














