matlab中slic实现
时间: 2023-09-30 16:06:07 浏览: 55
以下是一个简单的Matlab代码实现slic算法的示例:
```matlab
function [labels, centers] = slic(I, numSuperpixels, compactness)
% 参数设置
if nargin < 3
compactness = 20;
end
if nargin < 2
numSuperpixels = 1000;
end
% 初始化超像素中心点
[height, width, ~] = size(I);
step = sqrt(height * width / numSuperpixels);
x = round(step/2):round(step):width;
y = round(step/2):round(step):height;
[X, Y] = meshgrid(x, y);
centers = [X(:) Y(:)];
% 初始化超像素标签
labels = zeros(height, width);
labelIndex = 1;
% 迭代更新超像素中心点和标签
while true
newLabels = zeros(height, width);
for i = 1:size(centers, 1)
center = centers(i,:);
xStart = max(center(1) - 2*step, 1);
xEnd = min(center(1) + 2*step, width);
yStart = max(center(2) - 2*step, 1);
yEnd = min(center(2) + 2*step, height);
patch = I(yStart:yEnd, xStart:xEnd, :);
[patchHeight, patchWidth, ~] = size(patch);
[patchX, patchY] = meshgrid(xStart:xEnd, yStart:yEnd);
patchPoints = [patchX(:) patchY(:)];
patchColors = reshape(patch, patchHeight*patchWidth, []);
patchDistances = sum(bsxfun(@minus, patchColors, center).^2, 2);
patchLabels = labelIndex * ones(patchHeight*patchWidth, 1);
[~, idx] = min(patchDistances);
patchLabels(idx) = 0;
newLabels(yStart:yEnd, xStart:xEnd) = reshape(patchLabels, patchHeight, patchWidth);
end
% 计算超像素中心点的更新值
newCenters = zeros(size(centers));
for i = 1:size(centers, 1)
center = centers(i,:);
xStart = max(center(1) - 2*step, 1);
xEnd = min(center(1) + 2*step, width);
yStart = max(center(2) - 2*step, 1);
yEnd = min(center(2) + 2*step, height);
patchLabels = newLabels(yStart:yEnd, xStart:xEnd);
[patchX, patchY] = meshgrid(xStart:xEnd, yStart:yEnd);
patchPoints = [patchX(:) patchY(:)];
patchColors = reshape(I(yStart:yEnd, xStart:xEnd, :), [], 3);
patchLabels = patchLabels(:);
patchPoints = double(patchPoints);
patchColors = double(patchColors);
patchCenters = accumarray(patchLabels+1, [patchPoints patchColors], [labelIndex+1 8], @sum);
patchSizes = accumarray(patchLabels+1, ones(size(patchLabels)));
patchCenters(:,1:2) = patchCenters(:,1:2) ./ patchSizes;
patchCenters(:,3:5) = patchCenters(:,3:5) ./ patchCenters(:,8);
patchCenters(:,6:8) = sqrt(patchCenters(:,6:8) ./ patchSizes - patchCenters(:,3:5).^2);
patchCenters(2:end,6:8) = patchCenters(2:end,6:8) * compactness;
[~, idx] = min(sum((patchCenters(2:end,3:5) - repmat(center, labelIndex, 1)).^2, 2));
newCenters(i,:) = patchCenters(idx+1,1:2);
end
% 判断是否收敛
if sum(sum(newLabels ~= labels)) < 0.1 * height * width
break;
end
% 更新超像素中心点和标签
labels = newLabels;
centers = newCenters;
labelIndex = labelIndex + 1;
end
% 调整超像素标签编号
[~, ~, labels] = unique(labels);
labels = reshape(labels, height, width);
end
```
在这个示例中,我们首先定义了一个名为slic的函数,它接受三个输入参数:I为原始图像,numSuperpixels为期望得到的超像素数目,compactness为控制超像素形状的参数。如果用户没有提供这些参数,则使用默认值。然后,我们初始化了超像素中心点和标签,并开始迭代更新它们。在每次迭代中,我们首先使用当前的超像素中心点对图像进行分割,得到新的超像素标签。然后,我们计算新的超像素中心点的位置,以便下一次迭代使用。最后,我们检查当前的超像素标签是否与上一次迭代相同,如果是,则认为算法已经收敛,退出循环。最后,我们将超像素标签进行重新编号,以便它们可以被更方便地使用。
请注意,这个示例代码只是slic算法的一种简单实现,可能不够高效或准确。实际使用时应该根据具体情况进行调整和优化。
相关推荐
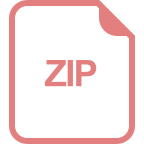














