java中判断字符串是否为空
时间: 2024-09-12 12:16:50 浏览: 19
在Java中,可以使用多种方式来判断一个字符串是否为空:
1. **直接比较**:使用`isEmpty()`方法,如果字符串长度为0,则认为为空。
```java
String str = "";
if (str.isEmpty()) {
System.out.println("字符串为空");
}
```
2. **null检查**:如果字符串变量未赋值或者为null,也表示为空。
```java
String str = null;
if (str == null) {
System.out.println("字符串为空");
}
```
3. **使用三元运算符**:
```java
String str = "hello";
boolean isNullOrEmpty = str == null || str.length() == 0;
if (isNullOrEmpty) {
System.out.println("字符串为空");
}
```
4. **使用equals()方法**:虽然这不是严格意义上的空检查,但在某些场景下,可能会检查字符串内容是否等于某个特定值,如"null"。
```java
if ("".equals(str)) {
System.out.println("字符串为空");
}
```
相关问题
Java怎么判断字符串是否为空
在Java中,可以使用以下方法判断字符串是否为空:
1. 使用`isEmpty()`方法:
```java
String str = "Hello";
if (str.isEmpty()) {
System.out.println("字符串为空");
} else {
System.out.println("字符串不为空");
}
```
2. 使用`length()`方法:
```java
String str = "Hello";
if (str.length() == 0) {
System.out.println("字符串为空");
} else {
System.out.println("字符串不为空");
}
```
3. 使用`equals()`方法:
```java
String str = "Hello";
if (str.equals("")) {
System.out.println("字符串为空");
} else {
System.out.println("字符串不为空");
}
```
4. 使用`isBlank()`方法(Java 11+):
```java
String str = "Hello";
if (str.isBlank()) {
System.out.println("字符串为空");
} else {
System.out.println("字符串不为空");
}
```
以上方法可以判断字符串是否为空。如果字符串为空,返回true;否则返回false。
判断字符串是否为空java
Java中判断字符串是否为空可以使用以下几种方法:
1. 使用String类的isEmpty()方法,判断字符串是否为空:
String str = "";
if (str.isEmpty()) {
System.out.println("字符串为空");
}
2. 使用String类的length()方法,判断字符串长度是否为0:
String str = "";
if (str.length() == 0) {
System.out.println("字符串为空");
}
3. 使用String类的trim()方法,去除字符串两端的空格后再判断是否为空:
String str = " ";
if (str.trim().isEmpty()) {
System.out.println("字符串为空");
}
4. 使用StringUtils类的isEmpty()方法,判断字符串是否为空:
import org.apache.commons.lang.StringUtils;
String str = "";
if (StringUtils.isEmpty(str)) {
System.out.println("字符串为空");
}
其中第四种方法需要引入org.apache.commons.lang库。
相关推荐
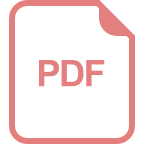
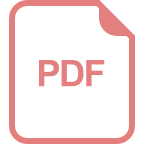
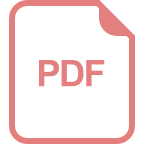












