个人网页也是fragment ,修改的网页也是fragment ,修改和返回显示的代码
时间: 2024-03-31 16:33:48 浏览: 40
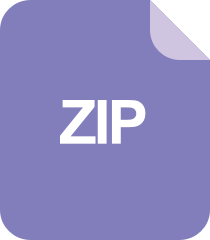
Fragment总结共15页.pdf.zip
假设个人网页和修改页面都是 Fragment,并且它们是在同一个 Activity 中进行切换和显示的。以下是一个简单的示例代码,可以实现在修改页面中修改个人信息并在个人网页中显示更新后的信息:
在 Activity 中定义两个 Fragment:
```java
public class ProfileFragment extends Fragment {
private TextView usernameTextView;
private TextView emailTextView;
// onCreateView 方法用于创建 Fragment 的视图
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// 加载布局文件
View view = inflater.inflate(R.layout.fragment_profile, container, false);
// 获取用户名和邮箱的 TextView
usernameTextView = view.findViewById(R.id.usernameTextView);
emailTextView = view.findViewById(R.id.emailTextView);
// 显示初始的个人信息
showProfile();
return view;
}
// 显示个人信息的方法
private void showProfile() {
// 从后端服务器或本地存储中获取个人信息
String username = "Alice";
String email = "alice@example.com";
// 更新用户名和邮箱的 TextView
usernameTextView.setText(username);
emailTextView.setText(email);
}
}
public class EditProfileFragment extends Fragment {
private EditText usernameEditText;
private EditText emailEditText;
// onCreateView 方法用于创建 Fragment 的视图
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// 加载布局文件
View view = inflater.inflate(R.layout.fragment_edit_profile, container, false);
// 获取用户名和邮箱的 EditText
usernameEditText = view.findViewById(R.id.usernameEditText);
emailEditText = view.findViewById(R.id.emailEditText);
// 显示初始的个人信息
showProfile();
// 获取保存按钮并添加点击事件
Button saveButton = view.findViewById(R.id.saveButton);
saveButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
saveProfile();
// 切换回个人网页
getFragmentManager().popBackStack();
}
});
return view;
}
// 显示个人信息的方法
private void showProfile() {
// 从后端服务器或本地存储中获取个人信息
String username = "Alice";
String email = "alice@example.com";
// 更新用户名和邮箱的 EditText
usernameEditText.setText(username);
emailEditText.setText(email);
}
// 保存个人信息的方法
private void saveProfile() {
// 获取修改后的用户名和邮箱
String username = usernameEditText.getText().toString();
String email = emailEditText.getText().toString();
// 将个人信息保存到后端服务器或本地存储中
// ...
// 更新个人网页中的用户名和邮箱
ProfileFragment profileFragment = (ProfileFragment) getFragmentManager()
.findFragmentById(R.id.profileFragment);
profileFragment.showProfile();
}
}
```
在 Activity 的布局文件中添加两个 Fragment 的占位符:
```xml
<FrameLayout
android:id="@+id/fragmentContainer"
android:layout_width="match_parent"
android:layout_height="match_parent" />
```
在 Activity 中使用 FragmentTransaction 来切换和显示 Fragment:
```java
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// 初始显示个人网页
ProfileFragment profileFragment = new ProfileFragment();
getSupportFragmentManager().beginTransaction()
.replace(R.id.fragmentContainer, profileFragment)
.commit();
// 点击修改按钮切换到修改页面
Button editButton = findViewById(R.id.editButton);
editButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
EditProfileFragment editProfileFragment = new EditProfileFragment();
getSupportFragmentManager().beginTransaction()
.replace(R.id.fragmentContainer, editProfileFragment)
.addToBackStack(null)
.commit();
}
});
}
}
```
在 EditProfileFragment 中,点击保存按钮后,会先调用 saveProfile 方法保存个人信息并更新后端服务器或本地存储中的信息,然后使用 popBackStack 方法切换回个人网页。在 saveProfile 方法中,还会获取个人网页的 Fragment 并调用其 showProfile 方法来更新个人网页中的信息。
需要注意的是,以上代码仅为示例代码,具体实现方式会因应用的需求和架构而有所差异。
阅读全文
相关推荐
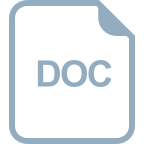
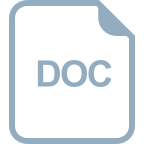
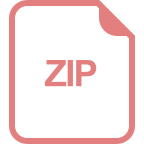
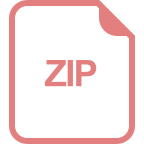
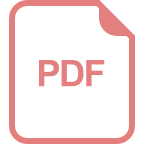
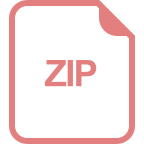
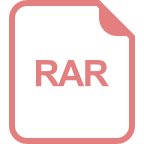
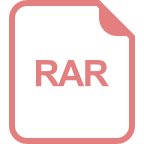
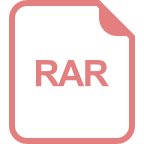
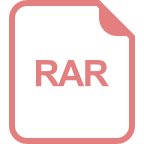
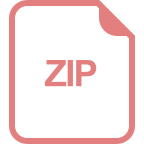
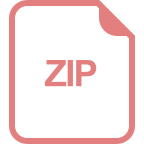
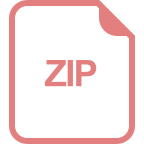
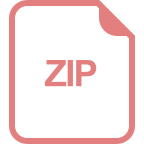


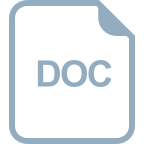
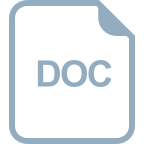
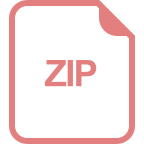