qt解析json中的树结构和列表
时间: 2024-03-23 08:39:00 浏览: 34
在 Qt 中解析 JSON 树结构和列表可以使用 QJsonDocument 和 QJsonObject 类来实现。
1. 解析 JSON 树结构:
假设有以下 JSON 数据:
```
{
"name": "John",
"age": 30,
"children": [
{
"name": "Alice",
"age": 5
},
{
"name": "Bob",
"age": 3
}
]
}
```
代码示例:
```cpp
QJsonDocument doc = QJsonDocument::fromJson(jsonData.toUtf8());
QJsonObject obj = doc.object();
QString name = obj.value("name").toString();
int age = obj.value("age").toInt();
QJsonArray childrenArray = obj.value("children").toArray();
foreach (const QJsonValue &value, childrenArray) {
QJsonObject childObj = value.toObject();
QString childName = childObj.value("name").toString();
int childAge = childObj.value("age").toInt();
// do something with childName and childAge
}
```
2. 解析 JSON 列表:
假设有以下 JSON 数据:
```
[
{
"name": "John",
"age": 30
},
{
"name": "Alice",
"age": 5
},
{
"name": "Bob",
"age": 3
}
]
```
代码示例:
```cpp
QJsonDocument doc = QJsonDocument::fromJson(jsonData.toUtf8());
QJsonArray array = doc.array();
foreach (const QJsonValue &value, array) {
QJsonObject obj = value.toObject();
QString name = obj.value("name").toString();
int age = obj.value("age").toInt();
// do something with name and age
}
```
注意:在解析 JSON 数据时,需要确保 JSON 数据的格式正确。如果格式不正确,解析过程可能会出现异常。
相关推荐
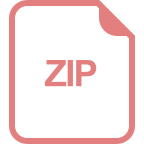
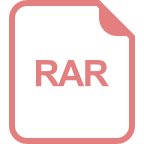














