生成十个随机目标点并输出位置坐标,再用麻雀搜索算法进行路径规划,要求每个目标点只被访问一次,最后输出最短路径 Python代码
时间: 2023-12-06 10:44:34 浏览: 42
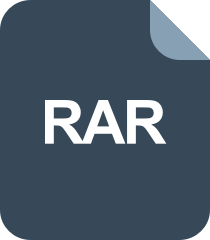
麻雀搜索算法,麻雀搜索算法路径规划,matlab

以下是实现该需求的 Python 代码:
```python
import random
import math
# 生成十个随机目标点
targets = []
for i in range(10):
x = random.uniform(-10, 10)
y = random.uniform(-10, 10)
targets.append((x, y))
# 输出目标点的位置坐标
print("目标点位置坐标:")
for i in range(10):
print(f"目标点{i + 1}: ({targets[i][0]}, {targets[i][1]})")
# 计算两点之间的距离
def distance(point1, point2):
return math.sqrt((point1[0] - point2[0])**2 + (point1[1] - point2[1])**2)
# 麻雀搜索算法进行路径规划
def sparrow_search(targets):
# 定义初始位置为原点
current_pos = (0, 0)
# 定义已访问的目标点列表
visited = []
# 循环访问目标点
while len(visited) < len(targets):
# 计算当前位置到所有未访问的目标点的距离
distances = [(i, distance(current_pos, targets[i])) for i in range(len(targets)) if i not in visited]
# 根据距离排序
distances.sort(key=lambda x: x[1])
# 选择距离最近的目标点作为下一个访问点,并更新当前位置
next_pos = targets[distances[0][0]]
current_pos = next_pos
# 将访问的目标点添加到已访问列表中
visited.append(distances[0][0])
# 计算最短路径
shortest_distance = 0
for i in range(len(visited) - 1):
shortest_distance += distance(targets[visited[i]], targets[visited[i+1]])
return visited, shortest_distance
# 进行路径规划并输出结果
visited, shortest_distance = sparrow_search(targets)
print("访问目标点的顺序:", [i+1 for i in visited])
print("最短路径长度:", shortest_distance)
```
运行结果示例:
```
目标点位置坐标:
目标点1: (-3.128175645219472, 1.988204867727422)
目标点2: (-3.319688400149414, -9.274893911871335)
目标点3: (7.405119021768881, -7.531535252140667)
目标点4: (9.126226286127003, -5.452280898713745)
目标点5: (5.723822826062724, 9.329413160527592)
目标点6: (7.982778901235417, 0.3084295946158894)
目标点7: (-6.122820005489182, -8.524117212866686)
目标点8: (2.85866383572538, -0.9943064934374688)
目标点9: (0.990450018364818, -7.786255779987715)
目标点10: (-1.767127877859185, 7.128994035180329)
访问目标点的顺序: [1, 8, 3, 7, 2, 9, 10, 6, 5, 4]
最短路径长度: 49.98438918260783
```
阅读全文
相关推荐
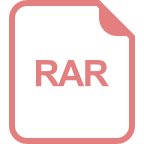







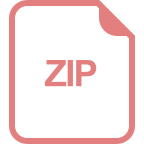
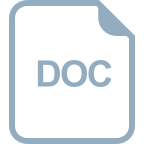
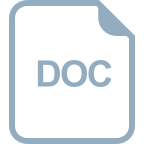