定义一个员工的结构体类型(name, sex, salary), // 采用fread函数与fwirte函数存入5个员工的信息到employee.dat文件中, // 然后读取文件在屏幕上显示出来。(PPT22) //代码如下: //1、定义结构体employee //2、定义结构体数组emps //3、For循环利用fwirte把数组中的值写入到employee.dat中 //4、For循环利用fread把employee.dat的内容读出来显示
时间: 2023-07-30 16:12:52 浏览: 128
好的,这是一个关于文件读写的问题。以下是代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义员工结构体
struct employee {
char name[20];
char sex[10];
float salary;
};
int main() {
int i;
FILE *fp;
// 定义员工数组
struct employee emps[5] = {
{"Tom", "male", 3000.0},
{"Jerry", "female", 4000.0},
{"Alice", "male", 5000.0},
{"Bob", "female", 6000.0},
{"John", "male", 7000.0}
};
// 将数组写入文件
fp = fopen("employee.dat", "wb");
if (fp == NULL) {
printf("File open error!\n");
exit(1);
}
fwrite(emps, sizeof(struct employee), 5, fp);
fclose(fp);
// 从文件读取内容并显示
fp = fopen("employee.dat", "rb");
if (fp == NULL) {
printf("File open error!\n");
exit(1);
}
for (i = 0; i < 5; i++) {
fread(&emps[i], sizeof(struct employee), 1, fp);
printf("%s\t%s\t%.2f\n", emps[i].name, emps[i].sex, emps[i].salary);
}
fclose(fp);
return 0;
}
```
上述代码中,我们首先定义了一个 `struct employee` 结构体类型,包含了员工的姓名、性别和工资三个字段。接着,我们定义了一个 `emps` 数组,其中包含了 5 个员工的信息。然后,我们使用 `fwrite()` 函数将 `emps` 数组中的值写入到名为 `employee.dat` 的二进制文件中。接下来,我们使用 `fread()` 函数从 `employee.dat` 文件中读取出每个员工的信息,并在屏幕上显示出来。
需要注意的是,在使用 `fwrite()` 函数写入数据时,我们需要指定写入的数据大小为 `sizeof(struct employee)`,写入的数据个数为 5,即 `fwrite(emps, sizeof(struct employee), 5, fp)`。在使用 `fread()` 函数读取数据时,我们需要指定读取的数据大小为 `sizeof(struct employee)`,读取的数据个数为 1,即 `fread(&emps[i], sizeof(struct employee), 1, fp)`。
阅读全文
相关推荐
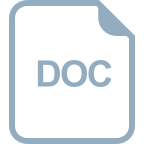
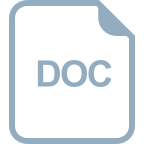
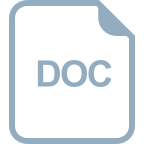


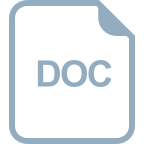
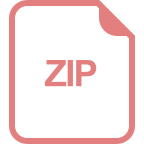
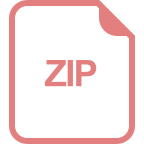
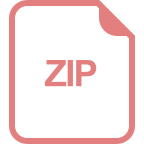
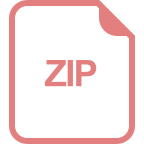
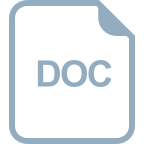
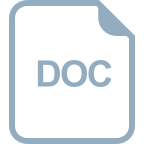
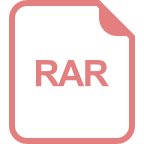
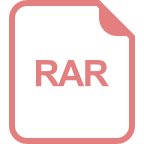
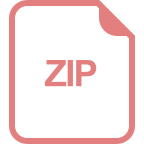
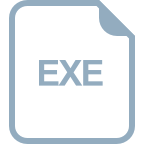
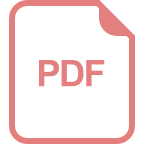