JS判断一个数组中存在某个字符串
时间: 2023-09-09 12:10:17 浏览: 96
可以使用数组的 includes() 方法来判断一个数组中是否存在某个字符串。例如:
```
var fruits = ["apple", "banana", "orange"];
console.log(fruits.includes("banana")); //true
console.log(fruits.includes("grape")); //false
```
这段代码将会输出 `true` 和 `false`,分别表示数组中是否存在 `"banana"` 和 `"grape"`。
相关问题
js判断数组是否包含某个字符串
在JavaScript中,判断数组是否包含某个字符串有多种方法:
1. 使用传统的for循环或for...of循环遍历数组,然后使用if语句判断是否包含该字符串。
2. 使用数组的indexOf方法,如果返回值大于-1,则表示数组中包含该字符串。
3. 使用数组的find方法,如果找到该字符串,则返回该字符串,否则返回undefined。
4. 使用数组的findIndex方法,如果找到该字符串,则返回该字符串在数组中的索引,否则返回-1。
举个例子,如果我们有一个字符串数组arr,想要判断其中是否包含字符串str,可以使用以下代码:
```
//方法一:使用传统for循环
for(let i=0; i<arr.length; i++) {
if(arr[i] === str) {
console.log("数组中包含该字符串"); break;
}
}
//方法二:使用indexOf方法
if(arr.indexOf(str) > -1) {
console.log("数组中包含该字符串");
}
//方法三:使用find方法
if(arr.find(item => item === str)) {
console.log("数组中包含该字符串");
}
//方法四:使用findIndex方法
if(arr.findIndex(item => item === str) > -1) {
console.log("数组中包含该字符串");
}
```
js判断字符串数组是否包含某个字符代码示例
在JavaScript中,我们可以使用Array的includes方法来判断一个字符串数组是否包含某个特定的字符,代码示例如下:
```
const strArr = ["apple", "banana", "orange"];
if (strArr.includes("apple")) {
console.log("该数组包含 'apple' 字符");
} else {
console.log("该数组不包含 'apple' 字符");
}
```
希望这个回答对你有所帮助!如果你有更多的问题,请随时问我。
阅读全文
相关推荐
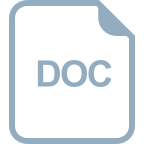
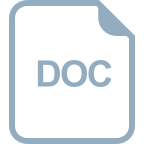
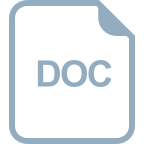
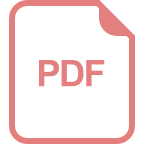












